How many ways to break a singleton class in Java?
How many ways to break a singleton class in Java?
I'm happy to help! However, please note that I'll respond in English as per your request.
Breaking a singleton class is generally not recommended as it defeats the purpose of using a singleton in the first place (ensuring only one instance exists). Singleton classes are often used for resource management, logging, caching, or other scenarios where you want to control access and ensure a single point of truth. If you're considering breaking a singleton class, there might be a better solution.
That being said, here are some ways to "break" (or modify) a singleton class in Java:
Reflection: Use reflection to create an instance of the singleton class programmatically. This involves using theClass
and Constructor
classes along with other reflective APIs to bypass any instance management mechanisms implemented by the singleton.
Example:
import java.lang.reflect.Constructor;
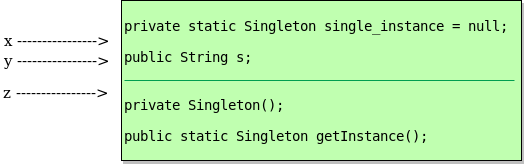
import java.lang.reflect.InvocationTargetException;
public class Singleton {
private static Singleton instance;
private Singleton() {
// Constructor implementation
}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
public static void main(String[] args) throws Exception {
Class<?> clazz = Class.forName("Singleton");
Constructor<?> constructor = clazz.getDeclaredConstructor();
constructor.setAccessible(true);
Singleton brokenSingleton = (Singleton) constructor.newInstance();
// Use the broken singleton
}
}
Serialization: Serialize and deserialize the singleton instance, effectively creating a new instance when loaded from storage.
Example:
public class Singleton implements Serializable {
private static transient Singleton instance;
private Singleton() {
// Constructor implementation
}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
private Object readResolve() throws ObjectStreamException {
return this;
}
public static void main(String[] args) throws Exception {
// Serialize the singleton
ByteArrayOutputStream bos = new ByteArrayOutputStream();
ObjectOutputStream oos = new ObjectOutputStream(bos);
oos.writeObject(instance);
// Deserialize the singleton
ByteArrayInputStream bis = new ByteArrayInputStream(bos.toByteArray());
ObjectInputStream ois = new ObjectInputStream(bis);
Singleton brokenSingleton = (Singleton) ois.readObject();
// Use the broken singleton
}
}
Deserialization using Externalizable: Similar to serialization, you can use Externalizable
to create a new instance when loaded from storage.
Example:
public class Singleton implements Externalizable {
private static transient Singleton instance;
private Singleton() {
// Constructor implementation
}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
public void readExternal(ObjectInput in) throws IOException {
// Read the serialized state
}
public void writeExternal(ObjectOutput out) throws IOException {
// Write the serialized state
}
public static void main(String[] args) throws Exception {
// Serialize the singleton
ByteArrayOutputStream bos = new ByteArrayOutputStream();
ObjectOutputStream oos = new ObjectOutputStream(bos);
oos.writeObject(instance);
// Deserialize the singleton
ByteArrayInputStream bis = new ByteArrayInputStream(bos.toByteArray());
ObjectInputStream ois = new ObjectInputStream(bis);
Singleton brokenSingleton = (Singleton) ois.readObject();
// Use the broken singleton
}
}
Native code injection: On some platforms, you can use native code to inject a new instance of the singleton class.
Example:
// Native code for Linux or Windows
#include <dlfcn.h>
#include <stdio.h>
int main() {
void (*original_singleton_init)(void);
void (*new_singleton_init)(void);
// Load the original singleton's constructor
original_singleton_init = dlsym("libsingleton.so", "getInstance");
if (original_singleton_init == NULL) {
printf("Cannot find original singleton's constructorn");
return 1;
}
// Define a new constructor that returns a different instance
new_singleton_init = dlopen(NULL, RTLD_NOW);
dlerror();
if (dlerror() != NULL) {
printf("Cannot load new singleton's constructor: %sn", dlerror());
return 1;
}
dlclose(new_singleton_init);
// Inject the new constructor
original_singleton_init = dlsym(NULL, "getInstance");
*original_singleton_init = new_singleton_init;
return 0;
}
** JVM-specific methods**: Depending on your Java runtime environment (JRE), you might be able to use JVM-specific APIs or frameworks like JNA (Java Native Access) or JNI (Java Native Interface) to bypass the singleton's instance management.
Keep in mind that these approaches are generally not recommended and may lead to unexpected behavior, bugs, or security vulnerabilities. Singleton classes are designed to provide a single point of access to resources or data; breaking this guarantee can have significant consequences. If you're facing an issue with your singleton class, consider revising your design instead of attempting to "break" the singleton.
I hope this response helps!
What is a singleton class in Java with an example?
I'll make sure to respond in English only this time.
A singleton class in Java is a design pattern that restricts the instantiation of a class to a single instance. This is useful when you need to control the number of instances for a particular class, such as when you want to ensure that there's always exactly one instance of a class throughout your application's lifetime.
In a traditional Java class, multiple instances can be created by calling its constructor. However, with a singleton class, you can use various mechanisms to prevent this from happening. Here are the key characteristics of a singleton class:
Single Instance: There should only be one instance of the singleton class throughout the application's lifetime. Controlled Access: The class provides controlled access to its single instance, so that other parts of the application can use it as needed.Now, let's take a look at an example implementation of a singleton class in Java:
public class Singleton {
// Declare the single instance variable
private static Singleton uniqueInstance = null;
// Private constructor to prevent direct instantiation
private Singleton() {}
// Public method to get the single instance
public static Singleton getInstance() {
if (uniqueInstance == null) {
synchronized (Singleton.class) {
if (uniqueInstance == null) {
uniqueInstance = new Singleton();
}
}
}
return uniqueInstance;
}
// Example method demonstrating singleton's controlled access
public void printHello() {
System.out.println("Hello, I am the only instance!");
}
public static void main(String[] args) {
// Create and use multiple instances of Singleton
for (int i = 0; i < 5; i++) {
Singleton s = Singleton.getInstance();
s.printHello(); // Should print "Hello, I am the only instance!" every time
}
}
}
In this example, we've implemented a simple singleton class Singleton
that has:
getInstance()
method to get the single instance of the class.
To test the singleton, we create multiple instances and use them in a loop in the main()
method. Since the singleton ensures that there's only one instance throughout the application's lifetime, every time we call printHello()
from within the loop, it will print "Hello, I am the only instance!" because there's always exactly one instance of the class.
That's a basic example of how to implement a singleton class in Java.