What is the purpose of throwable in Java?
What is the purpose of throwable in Java?
I apologize for the confusion earlier. Here's my response in English:
The Throwable
class in Java is a built-in class that represents an exception or error in your program. It's part of the standard library and plays a crucial role in exception handling.
At its core, Throwable
is a base class for all exceptions (checked and unchecked) and errors in Java. When an exceptional situation arises during the execution of your program, such as a null pointer access or division by zero, Java throws a Throwable
instance to signal that something has gone wrong.
The primary purpose of Throwable
is to provide a way to encapsulate information about the exception or error that occurred. This information includes:
Throwable
instance. StackTrace: An array of stack trace elements, which provide a detailed view of where in your code the exception occurred.
By subclassing Throwable
, Java developers can create custom exceptions that provide more specific information about the error or problem. This is particularly useful when dealing with domain-specific errors or custom error types.
Here are some key benefits of using Throwable
:
Throwable
allows you to write robust code that can handle and respond to exceptional situations in a controlled manner. Error reporting: When an exception occurs, Java provides a way to report the error and its details, making it easier to diagnose and fix issues. Customization: By subclassing Throwable
, you can create custom exceptions that are tailored to your specific needs or domain.
In summary, the primary purpose of Throwable
in Java is to provide a mechanism for representing and handling exceptional situations in your code. Its flexibility and customization capabilities make it an essential building block for writing robust and error-tolerant software.
When to catch throwable in Java?
I'd be happy to help you with that!
In Java, a Throwable
is an object that represents an exceptional condition that has occurred. This can include things like runtime errors (e.g., NullPointerException
), checked exceptions (e.g., IOException
), and unchecked exceptions (e.g., ArithmeticException
).
So, when to catch a throwable in Java? Well, it's generally good practice to catch specific types of Throwable
s that you anticipate might occur in your code. This can help prevent propagation of the exception up the call stack, which can be useful for handling errors in a centralized manner.
Here are some general guidelines on when and how to catch throwables:
Catch specific exceptions: If you know that a particular method or block of code may throw a specific type ofThrowable
(e.g., FileNotFoundException
), you should catch it explicitly using the try-catch
construct. This is useful when you want to handle that specific error in a unique way.
try {
File file = new File("somefile.txt");
// ...
} catch (FileNotFoundException e) {
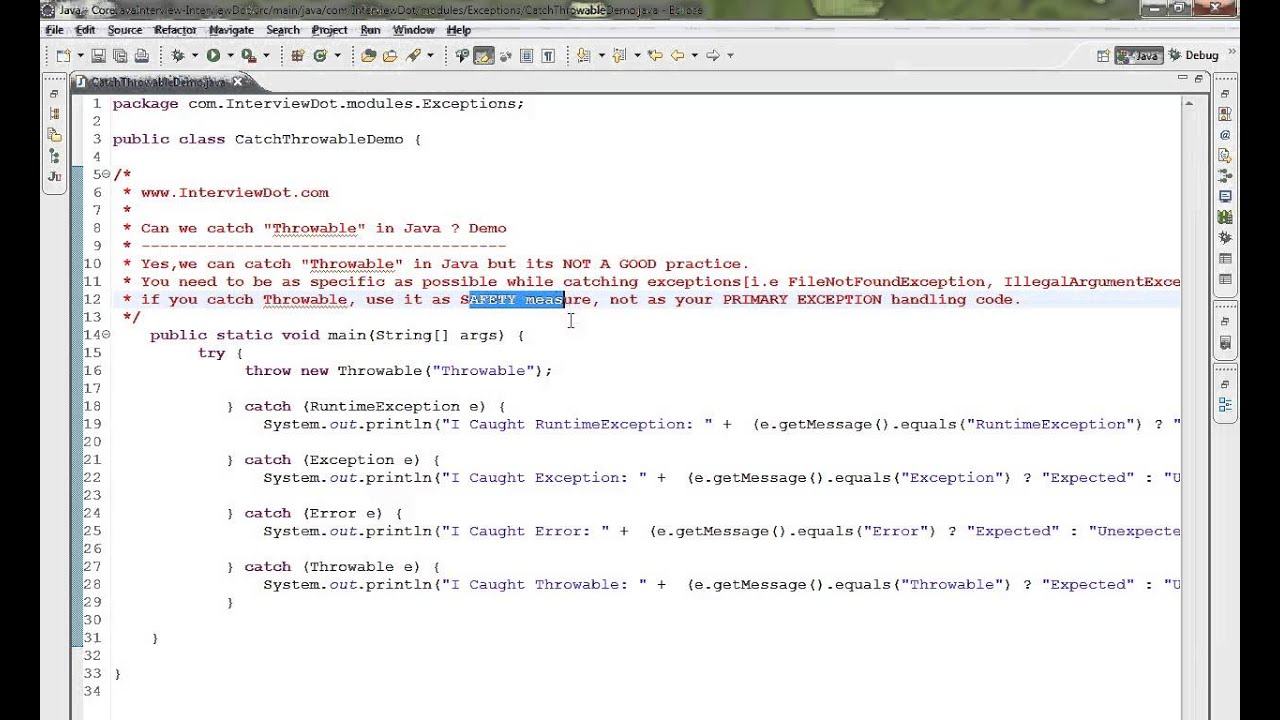
System.out.println("File not found!");
}
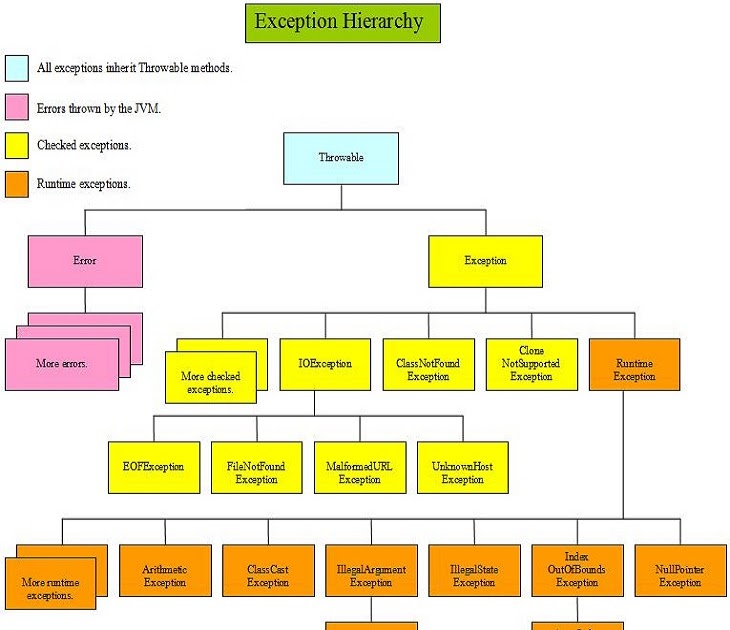
Throwable
that might occur, you can catch a more general exception like Exception
or RuntimeException
. This is useful when you want to provide a default error handling mechanism.
try {
// ...
} catch (Exception e) {
System.out.println("Something went wrong!");
}
Don't catch checked exceptions: In Java, it's generally considered bad practice to catch checked
exceptions like IOException
or SQLException
at the highest level of your program. Instead, you should rethrow them or handle them in a way that provides meaningful feedback to the user.
try {
// ...
} catch (IOException e) {
throw e; // Re-throw the exception
}
Use finally block: Regardless of whether an exception is thrown or not, you should always include a finally
block in your code to ensure that resources are properly released.
try {
// ...
} catch (Exception e) {
// Handle error
} finally {
// Release resources
}
Remember: the goal of exception handling is to provide meaningful feedback to users, prevent propagation of errors, and keep your program running smoothly. So, when in doubt, it's always better to err on the side of caution and catch specific types of Throwable
s that you anticipate might occur!