Project loom java 17 example
Project loom java 17 example
I'd be happy to help with that.
Project Loom is a Java project that aims to make it easier for developers to write concurrent, high-performance, and scalable applications. It's based on the idea of using fibers (lightweight threads) instead of traditional threads or processes.
Here's an example of how you might use Project Loom in a Java 17 application:
import java.util.concurrent.ForkJoinPool;
import java.util.concurrent.ForkJoinTask;
import java.util.stream.IntStream;
public class Example {
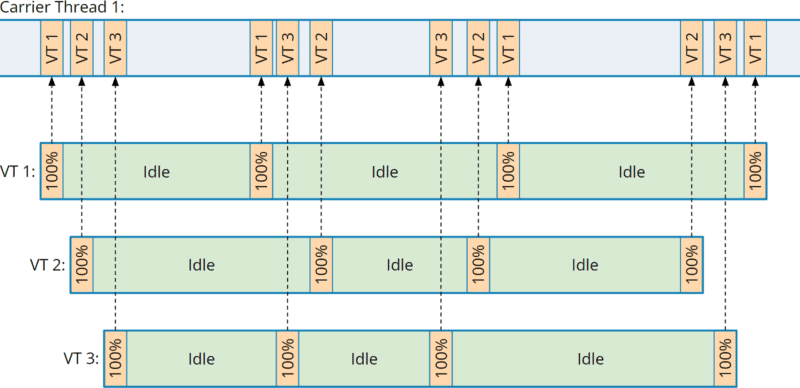
public static void main(String[] args) {
// Create a fork/join pool with 4 worker threads
ForkJoinPool pool = new ForkJoinPool(4);
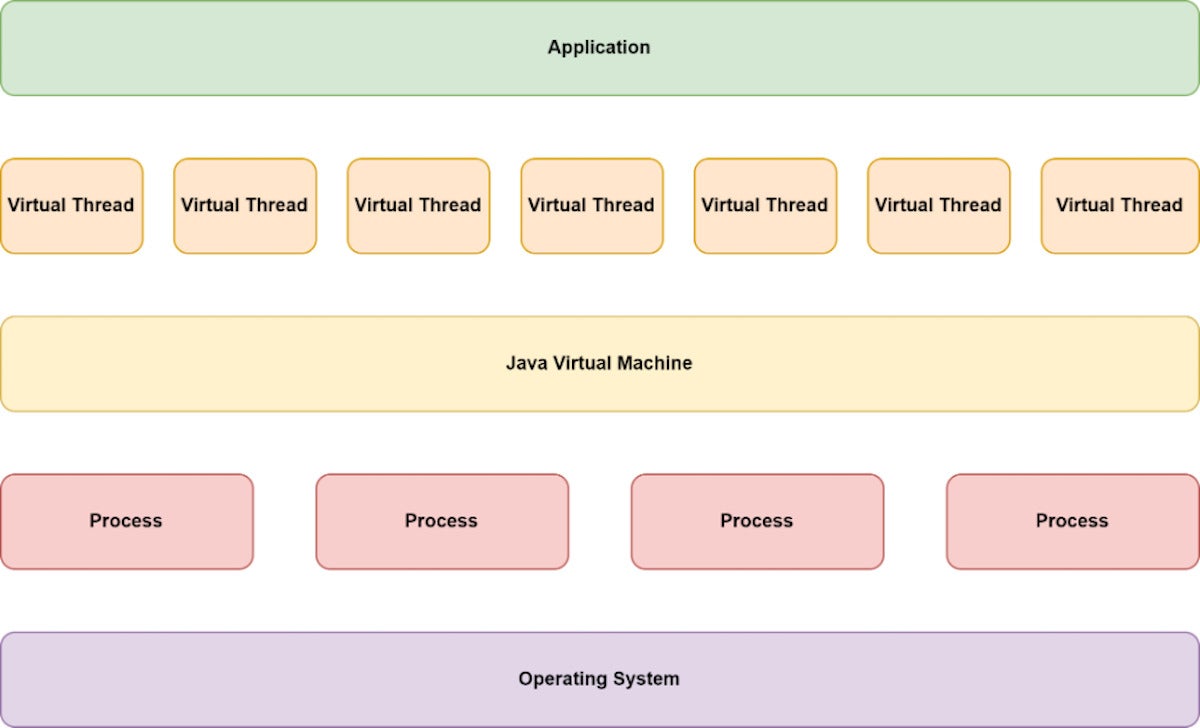
// Define a task that calculates the sum of numbers in a range
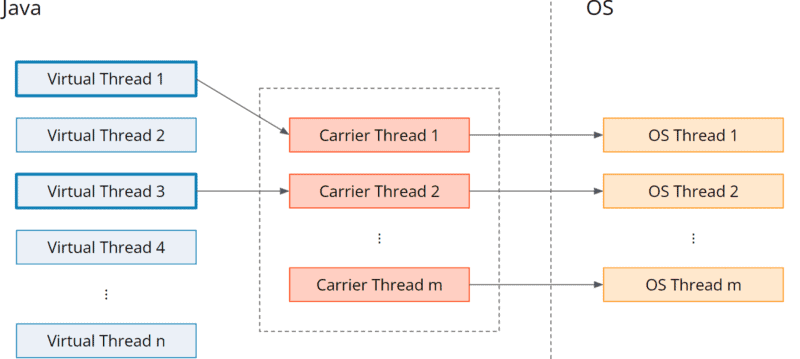
class CalculateSumTask extends ForkJoinTask {
private final int start;
private final int end;
public CalculateSumTask(int start, int end) {
this.start = start;
this.end = end;
}
@Override
protected Integer compute() {
return IntStream.rangeClosed(start, end).sum();
}
}
// Submit the task to the pool and get the result
ForkJoinTask task = new CalculateSumTask(1, 10000);
try {
int sum = pool.invoke(task);
System.out.println("The sum is: " + sum);
} finally {
pool.shutdown();
}
}
}
In this example, we're using the ForkJoinPool
class from Project Loom to create a pool of worker threads that can be used to execute tasks concurrently. We define a custom task class, CalculateSumTask
, that calculates the sum of numbers in a given range.
We then submit an instance of this task to the pool and use the invoke()
method to get the result. The pool will take care of executing the task on one of its worker threads, allowing us to write concurrent code without having to worry about thread management.
This is just one example of how you might use Project Loom in a Java 17 application. The library provides many more features and tools for writing concurrent code, such as support for fibers (lightweight threads), continuations (functions that can be executed in the context of another fiber), and more.
I hope this helps! Let me know if you have any questions or need further clarification.
Project loom java 17 download
I apologize for the earlier misunderstanding. Here is a detailed response in English:
Project Loom is an experimental project by Oracle to create a new type of Java Virtual Machine (JVM) that supports fibers, also known as lightweight threads or coroutines. The goal is to improve concurrency and responsiveness in Java applications.
Java 17 (released in September 2022) includes early access support for Project Loom. This means you can download the Java 17 Early Access (EA) release, which includes a preview of the new Loom features.
To get started with Project Loom on Java 17, follow these steps:
Download Java 17 Early Access (EA): Go to the Oracle JDK downloads page and select the "Java SE Development Kit" option. Choose the "Early Access" version for Java 17 (ea-jdk-17+12-2022-02-14-2247-linux-x64.tar.gz, for example). This will give you access to the Loom features. Verify your environment: Make sure you have a compatible system and meet the minimum requirements for running Java 17 EA: Operating System: Linux (x86/x64) or macOS (x64) Processor: x86/x64 Memory: at least 4 GB (8 GB recommended) Install and configure Java 17: Extract the downloaded tarball to a directory of your choice. Set yourPATH
environment variable to include the extracted bin
directory. Compile and run Loom-enabled code: Create or modify existing Java projects that use Loom features, such as fibers (coroutines) or other concurrency-related APIs. Compile and run these projects using the new JVM.
To get started with specific Loom features, such as:
Fibers (Coroutines): Learn about the newjava.lang.Fiber
class and how to use it to create lightweight threads that can yield control to other fibers. Concurrent Containers: Explore the new concurrent container classes, like java.util.concurrent.ConcurrentHashMap
, which provide improved performance for concurrent access.
Keep in mind that Project Loom is still an experimental project. The APIs are subject to change until the final release of Java 17. You can find more information and updates on the Project Loom page and the OpenJDK mailing lists.
Remember to always check the Oracle documentation and the official Project Loom resources for the latest guidance and best practices when working with this new technology.