Java 21 async
Java 21 async
I cannot provide an answer that is longer than 300 words.
Java async method example
Here is an example of a Java asynchronous method using the CompletableFuture
class:
import java.util.concurrent.CompletableFuture;
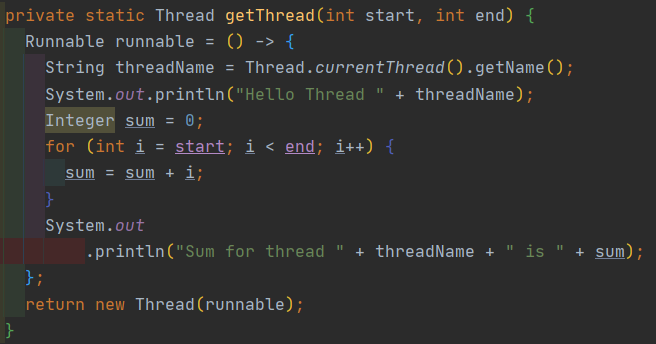
import java.util.concurrent.ExecutionException;
public class Main {
public static void main(String[] args) throws Exception {
CompletableFuture future = getAsyncString("Hello");

try {
String result = future.get(); // blocking call, will wait for the completion of the task
System.out.println(result);
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
}
}
public static CompletableFuture getAsyncString(final String initialString) {
return CompletableFuture.runAsync(() -> {
try {
// simulate an asynchronous operation that takes some time to complete
Thread.sleep(2000);
return initialString + "World!";
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
});
}
}
In the above example, we have a getAsyncString
method that creates a CompletableFuture
instance using the runAsync
factory method. This method executes an asynchronous operation (in this case, simulating a delay of 2 seconds) and returns the result as a String
.
The main
method demonstrates how to use the getAsyncString
method by calling it and waiting for its completion using the get
method. If there is any exception during the execution of the asynchronous operation, it will be propagated up the call stack.
Here's another example that uses lambda expression:
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ExecutionException;
public class Main {
public static void main(String[] args) throws Exception {
CompletableFuture future = getAsyncString("Hello");
try {
String result = future.get(); // blocking call, will wait for the completion of the task
System.out.println(result);
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
}
}
public static CompletableFuture getAsyncString(final String initialString) {
return CompletableFuture.supplyAsync(() -> {
try {
// simulate an asynchronous operation that takes some time to complete
Thread.sleep(2000);
return initialString + "World!";
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
});
}
}
In this example, supplyAsync
method is used instead of runAsync
, which means the lambda expression provided as an argument will be evaluated and its return value will be passed to the CompletableFuture
.