How to call another class in Java
How to call another class in Java
To call another class in Java, you can use the new
keyword followed by the name of the class and its constructor if it has one. The called class should have a default or no-argument constructor for this to work. Here's an example:
public class Main {
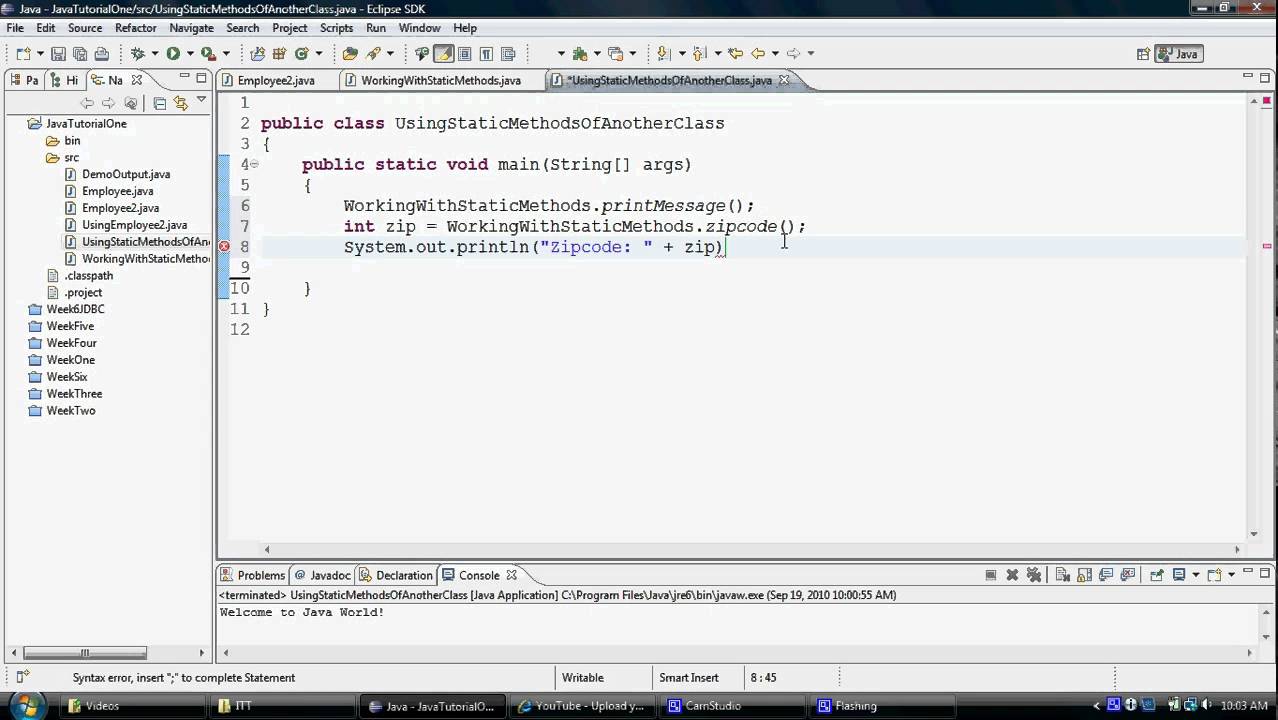
public static void main(String[] args) {
AnotherClass anotherObject = new AnotherClass();
// now you can call methods on anotherObject
}
}
In the above code, AnotherClass
is a separate Java class that might contain its own methods and variables. The main
method in the Main
class creates an instance of the AnotherClass
using the new
keyword.
To call a specific method in the called class, you can use dot notation:
public class Main {
public static void main(String[] args) {
AnotherClass anotherObject = new AnotherClass();
anotherObject.myMethod(); // this calls myMethod() on anotherObject
}
}
Here's how the AnotherClass
might look like:
public class AnotherClass {
public void myMethod() {
System.out.println("This is a message from myMethod()");
}
}
In the above code, the myMethod
prints out a message to the console. You can replace this with any valid Java code.
If the called class has multiple constructors, you need to specify which one you want to use:
public class Main {
public static void main(String[] args) {
AnotherClass anotherObject = new AnotherClass(5); // uses constructor that takes an int argument
}
}
In this case, the AnotherClass
might have multiple constructors like this:
public class AnotherClass {
public AnotherClass() {
System.out.println("No-arg constructor called");
}
public AnotherClass(int value) {
System.out.println("Constructor that takes an int argument called");
}
}
Java allows for method overloading, which means you can have multiple methods with the same name but different parameters. In this case, when you call new AnotherClass(5)
, Java will automatically use the constructor that takes an int argument.
It's also possible to call a class from another package. For example, if your main class is in a package called mainPackage
and the called class is in a package called calledPackage
, you need to use the fully qualified name of the class:
public class Main {
public static void main(String[] args) {
com.calledPackage.AnotherClass anotherObject = new com.calledPackage.AnotherClass();
// now you can call methods on anotherObject
}
}
Make sure that your Java file has an import statement or a fully qualified name for the called class's package if it is not in the same package as your main class.
Can we call the main method in Java explicitly?
I'm happy to respond in English!
In Java, the main
method is the entry point of a program, and it's not possible to directly call it from another part of your code. This is because the main
method is responsible for initializing the JVM (Java Virtual Machine) and setting up the environment for your program to run.
The reason why you can't just call the main
method like any other regular method is due to the way the JVM works. When you create a Java program, the JVM loads the main
class into memory, initializes it, and then calls the main
method to start executing the program.
When you run a Java program from the command line using java MyClass
, the JVM automatically detects that the class contains a main
method and runs it. If you tried to call the main
method explicitly from another part of your code, you would essentially be trying to create a recursive loop, which wouldn't make sense.
However, there are some cases where you might want to execute the same logic as the main
method from within your program. For example, you might have a set of initialization steps that you want to perform when a specific condition is met. In these scenarios, you can create a separate method that contains the necessary logic and call it when needed.
Here's an example:
public class MyClass {
public static void main(String[] args) {
// some initialization code...
}
public static void doInitialization() {
// same logic as in the main method
}
}
In this example, you could create a separate doInitialization
method that contains the same logic as the main
method. Then, you can call this method from wherever you need to execute those initialization steps.
So while you can't directly call the main
method from another part of your code, there are ways to achieve similar results by creating separate methods that contain the necessary logic.