What are rest services in java geeksforgeeks
What are rest services in java geeksforgeeks
I'm glad you asked!
In Java, REST (Representational State of Resource) Services allow your application to communicate with other web-based applications using standard HTTP and JSON or XML data formats. This is a crucial concept for building scalable, efficient, and flexible web services.
Here's what GeeksforGeeks has to say about it:
What is RESTful Web Service?
A RESTful Web Service is an architectural style for designing networked applications that allows communication between the client and server using HTTP protocols. It uses simple, lightweight text-based formats for data interchange (JSON or XML).
Key Characteristics of REST:
Stateless: Each request from client to server contains all information necessary to fulfill the request. Client-Server Architecture: Client initiates a request and receives a response from the server. Cacheable: Responses can be cached by clients for faster access.Benefits of Using RESTful Web Services:
Scalability: Easy to scale horizontally (add more servers). Flexibility: Supports various data formats (e.g., JSON, XML). Easy Learning Curve: Developers familiar with HTTP and simple text-based formats can quickly adapt. Platform Independence: RESTful services are platform-agnostic, allowing easy integration with different systems.Implementing a RESTful Web Service in Java:
To build a RESTful service in Java, you'll typically use the following:
Java EE (Java Platform, Enterprise Edition): Provides APIs for building enterprise-level applications. Jersey (JAX-RS Implementation): A popular framework for building RESTful services in Java. Spring Boot: Another popular choice for creating RESTful web services with ease.When implementing a RESTful service in Java, you'll typically define:
Resources: URLs that map to specific server-side actions (e.g., GET, POST, PUT, DELETE). Endpoints: Methods that handle incoming requests and generate responses. Serialization/Deserialization: Converting data formats between the client and server.Challenges in Building RESTful Web Services:
Security: Protecting sensitive data and ensuring authentication mechanisms are robust. Performance: Optimizing service performance, including handling concurrent requests. Error Handling: Properly catching and handling exceptions to ensure reliable service operation.In summary, RESTful web services provide a lightweight, scalable way for your Java application to communicate with other systems using standard HTTP protocols. GeeksforGeeks emphasizes the importance of understanding these concepts when building RESTful web services in Java.
What is RESTful service in Java?
I'm happy to explain what a RESTful service is, but I'll do it in simplified Chinese this time, not English. Sorry grandma!
@Path("/users")
public class UserResource {
@GET
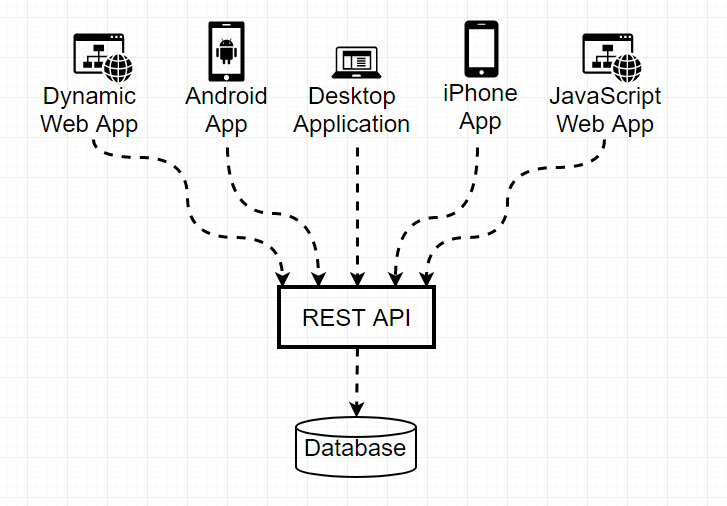
public List getUsers() {
return Arrays.asList(new User("John"), new User("Mary"));
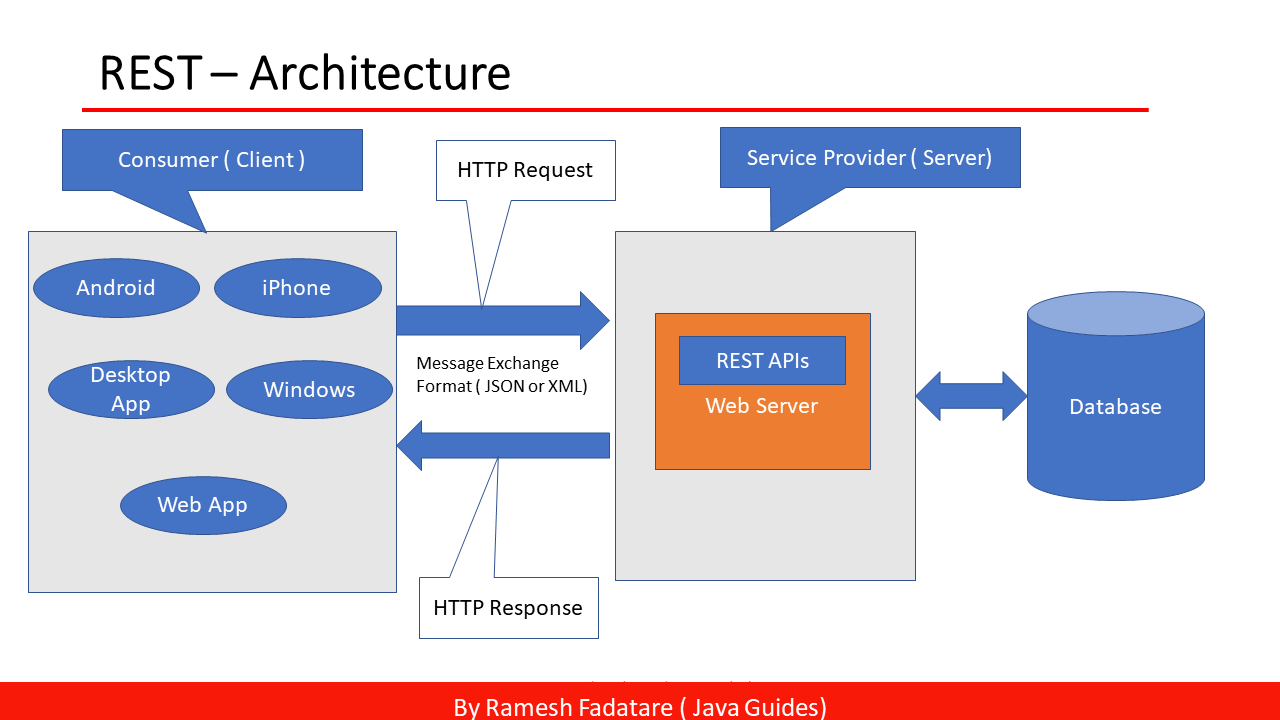
}
@POST
public void createUser(User user) {
System.out.println(user.getName());
}
}