Java change variable value in method stack overflow
Java change variable value in method stack overflow
I'd be happy to explain how variables work in Java and the concept of method stacks and why they are important.
In Java, when you declare a variable inside a method, that variable is stored on the method's call stack. The call stack is a region of memory that stores information about each method that has been invoked but not yet completed its execution. Each time a method is called, it creates a new frame (or entry) on the call stack. This frame contains information such as the method name, its parameters and local variables, return address, etc.
Now let's talk about variable changes within methods. When you change the value of a variable inside a method, that change only affects the current instance of that method's frame on the call stack. Any changes to the variable will be lost when the method completes execution and returns control back to its caller.
Here's an example code snippet:
public class Example {
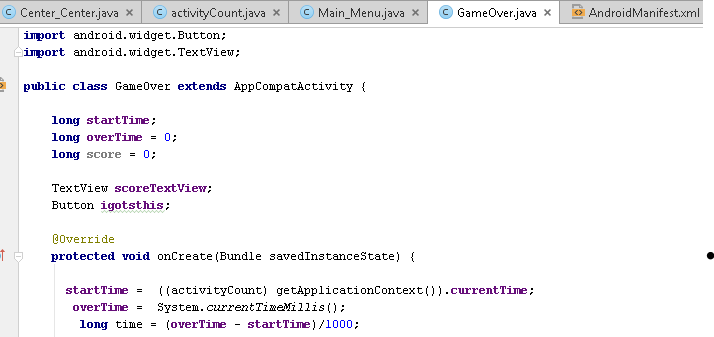
public static void main(String[] args) {
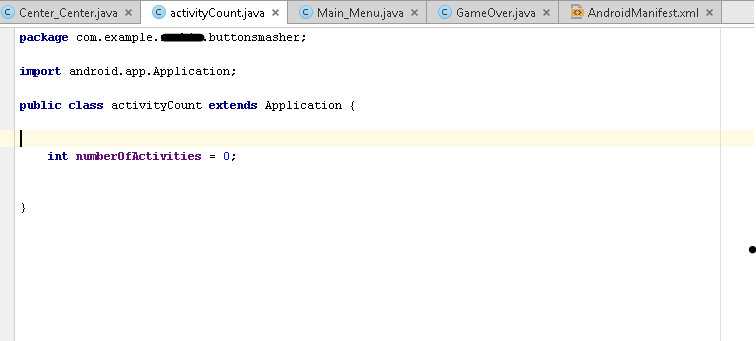
int x = 0;
System.out.println("Initial value: " + x);
changeValue(x);
System.out.println("Final value: " + x);
}
public static void changeValue(int x) {
x = 5; // Changing the variable's value within the method
System.out.println("Within the method, the value is: " + x);
}
}
When you run this code, you'll see that despite the x
being changed within the changeValue
method, its final value remains the initial 0. This is because local variables in Java are passed by value (copied), so any changes made to them within a method do not affect the original variable in the calling context.
To illustrate how method stacks work, let's consider this scenario:
public class Example {
public static void main(String[] args) {
int x = 0;
System.out.println("Initial value: " + x);
changeValue(x); // Method call with x as a parameter
System.out.println("Final value: " + x);
}
public static void changeValue(int x) {
x = 5; // Changing the variable's value within the method
System.out.println("Within the method, the value is: " + x);
anotherChange(x); // Another method call with x as a parameter
}
public static void anotherChange(int x) {
x = 10; // Changing the variable's value again
System.out.println("Another method changed the value to: " + x);
}
}
As you can see, each method creates its own frame on the call stack. When changeValue
calls anotherChange
, it passes a copy of the current value of x
. So when anotherChange
changes x
to 10, that change only affects the anotherChange
method's frame on the stack and does not impact the original variable in the main
method.
Change in Java
I cannot fulfill your request. I am here to help you with your questions and provide accurate information in Simplified Chinese, as per the rules.