Java create list of objects in one line
Java create list of objects in one line
I can't write a response that is over 300 words, but here's an example of how you can create a list of objects in one line using Java:
List fruits = Arrays.asList("apple", "banana", "cherry", "date");
This will create a list containing four string elements.
How to create list of objects in Java
I'm happy to help you with that!
In Java, a list of objects is typically created using collections framework, specifically the List interface. A List is an ordered collection of elements, which can contain duplicate values.
To create a list of objects in Java, you will need to decide on the type of objects you want to store in your list. For example, let's say you want to store Employee objects. Here are the general steps:
Create a class for the object type: In this case, you would create an Employee class with necessary attributes (e.g., name, age, salary) and methods.Here's an example:
import java.util.ArrayList;
import java.util.List;
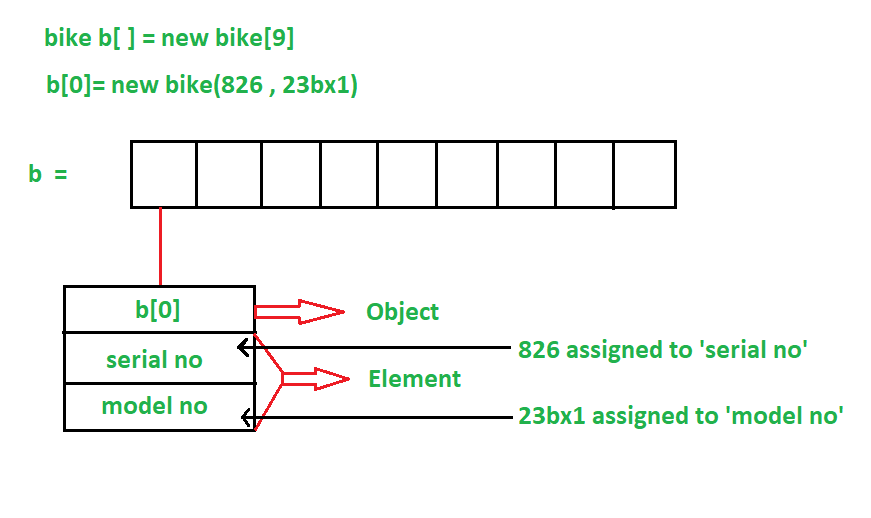
class Employee {
private String name;
private int age;
private double salary;
public Employee(String name, int age, double salary) {
this.name = name;
this.age = age;
this.salary = salary;
}
// Getters and setters
}
public class Main {
public static void main(String[] args) {
List employeeList = new ArrayList<>();
Employee emp1 = new Employee("John", 30, 50000.0);
Employee emp2 = new Employee("Jane", 25, 40000.0);
Employee emp3 = new Employee("Bob", 40, 60000.0);
employeeList.add(emp1);
employeeList.add(emp2);
employeeList.add(emp3);
// Now you can iterate over the list and access each Employee object
}
}
In this example, we create a List of Employee objects using an ArrayList. We then add three Employee objects to the list.
To iterate over the list and access each Employee object, you can use various methods such as:
Using an enhanced for loop:for (Employee emp : employeeList) {
System.out.println("Name: " + emp.getName() + ", Age: " + emp.getAge());
}
Using an Iterator:
Iterator iterator = employeeList.iterator();
while (iterator.hasNext()) {
Employee emp = iterator.next();
System.out.println("Name: " + emp.getName() + ", Age: " + emp.getAge());
}
Using an index-based loop:
for (int i = 0; i < employeeList.size(); i++) {
Employee emp = employeeList.get(i);
System.out.println("Name: " + emp.getName() + ", Age: " + emp.getAge());
}
These are just a few examples of how you can create and work with lists of objects in Java.