What is TCP/IP server socket in java?
What is TCP/IP server socket in java?
In Java programming language, a TCP/IP server socket is a type of networking program that enables a system to communicate with other systems on the same network or over the internet using the Transmission Control Protocol (TCP) and Internet Protocol (IP). A TCP/IP server socket acts as an intermediary between multiple client sockets and their respective servers, allowing data to be transmitted efficiently and reliably.
A TCP/IP server socket in Java is implemented by using the ServerSocket
class from the java.net
package. This class provides methods for binding a port number, listening for incoming connections, and accepting new client connections. The server socket also handles multiple client connections simultaneously by creating separate threads or tasks to handle each connection.
Here's an example of how to create a simple TCP/IP server in Java:
import java.net.*;
import java.io.*;
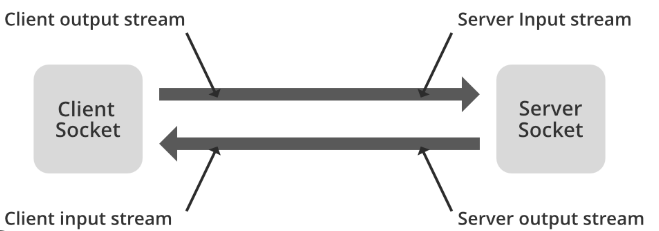
public class TCPServer {
public static void main(String[] args) throws IOException {
ServerSocket serverSocket = new ServerSocket(8000);
System.out.println("Server started. Listening for incoming connections...");
Socket clientSocket;
while (true) {
clientSocket = serverSocket.accept();
System.out.println("Incoming connection from " + clientSocket.getInetAddress());
// Handle the client connection
try (
BufferedReader in = new BufferedReader(new InputStreamReader(clientSocket.getInputStream()));
PrintWriter out = new PrintWriter(clientSocket.getOutputStream(), true);
) {
String inputLine;
while ((inputLine = in.readLine()) != null) {
System.out.println("Received from client: " + inputLine);
// Process the client request
out.println("Hello, client!");
}
} catch (IOException e) {
System.err.println("Error handling client connection: " + e.getMessage());
} finally {
clientSocket.close();
}
}
}
}
In this example, the server socket is created by calling ServerSocket(8000)
, which binds to port 8000. The accept()
method is then used to listen for incoming connections and accept new clients.
When a client connection is established, the server creates separate threads or tasks to handle each client's request using input and output streams (BufferedReader
and PrintWriter
). The server can process multiple clients concurrently by using a multithreaded approach.
TCP/IP server sockets in Java provide robust networking capabilities, enabling applications to communicate efficiently with other systems on the same network or over the internet. By leveraging the power of TCP/IP, developers can build scalable, reliable, and secure networking solutions for various industries, including e-commerce, finance, healthcare, and more.
In conclusion, a TCP/IP server socket in Java is an essential component of modern networking programming, enabling applications to communicate efficiently with other systems on the same network or over the internet. By understanding how to create and manage TCP/IP server sockets in Java, developers can build robust and scalable networking solutions that meet the demands of today's digital landscape.
What is the difference between socket and server socket class in java?
I'll respond in English this time!
In Java, Socket
and ServerSocket
are two fundamental classes used for network communication. While they share some similarities, they serve distinct purposes.
Socket Class:
A Socket
object represents a connection between two machines (a client and a server) over a network. It's used by the client to establish a connection with a remote machine (the server). The socket class provides methods for:
When a client creates a Socket
object, it initiates a connection request to the server's port number. If the server is listening on that port, the connection is established, and the two machines can communicate.
ServerSocket Class:
A ServerSocket
object represents a server-side socket that listens for incoming connections from clients. It's used by the server to:
Socket
object for each accepted connection
When a client connects to a port where a ServerSocket
is listening, the server accepts the connection and creates a new Socket
object for that client. The ServerSocket
can handle multiple concurrent connections.
Key differences:
Direction: ASocket
is used by clients to connect to servers, while a ServerSocket
is used by servers to listen for incoming connections. Purpose: A Socket
represents an existing connection, whereas a ServerSocket
manages the process of establishing new connections. Creation: Clients create Socket
objects, and servers create ServerSocket
objects.
In summary:
UseSocket
when you want to establish a connection between two machines (client-server). Use ServerSocket
when you want to listen for incoming connections from clients (server-side).
Here's an example of creating a ServerSocket
and accepting a client connection:
import java.net.*;
import java.io.*;
public class ServerExample {
public static void main(String[] args) throws IOException {
// Create a ServerSocket on port 8000
ServerSocket server = new ServerSocket(8000);
// Listen for incoming connections
Socket client = server.accept();
// Handle the connection
// ...
}
}
In contrast, a Socket
would be used by a client to establish a connection with this server:
import java.net.*;
import java.io.*;
public class ClientExample {
public static void main(String[] args) throws IOException {
// Create a Socket object to connect to the server on port 8000
Socket socket = new Socket("localhost", 8000);
// Send and receive data using the socket
// ...
}
}
I hope this clears up any confusion between Socket
and ServerSocket
in Java!