How to let user enter input in Java?
How to let user enter input in Java?
To allow users to input data in a Java program, you can use various methods depending on the type of application and the platform it runs on. Here are some common approaches:
Console Input: For console-based applications, you can use theScanner
class to read user input from the command line. The Scanner
class is part of the Java standard library, so you don't need to add any external libraries.
Here's an example:
import java.util.Scanner;
public class ConsoleInput {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter your name:");
String name = scanner.nextLine();
System.out.println("Hello, " + name + "!");
// Close the scanner to free up system resources
scanner.close();
}
}
In this example, System.in
represents the standard input stream, which is usually the keyboard. The nextLine()
method reads a line of text from the console and returns it as a string.
Here's an example using AWT:
import java.awt.Frame;
import java.awt.Label;
import java.awt.TextField;
public class GuiInput {
public static void main(String[] args) {
Frame frame = new Frame("My GUI App");
frame.setSize(300, 100);
Label label = new Label("Enter your name:");
frame.add(label);
TextField textField = new TextField();
frame.add(textField);
Button button = new Button("Submit");
button.addActionListener(e -> {
String input = textField.getText();
System.out.println("You entered: " + input);
});
frame.add(button, 2);
frame.setVisible(true);
}
}
In this example, a TextField
component is used to get user input. The getText()
method returns the text entered by the user.
TextInputControl
or TextFields
components to get user input.
Here's an example:
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.TextField;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class JavaFXInput extends Application {
@Override
public void start(Stage stage) {
TextField textField = new TextField();
textField.promptText("Enter your name:");
Button button = new Button("Submit");
button.setOnAction(e -> {
String input = textField.getText();
System.out.println("You entered: " + input);
});
VBox root = new VBox(10);
root.setPadding(new Insets(5));
root.getChildren().addAll(textField, button);
Scene scene = new Scene(root, 300, 100);
stage.setScene(scene);
stage.show();
}
}
In this example, a TextField
component is used to get user input. The promptText()
method sets the text prompt for the field.
Here's an example using servlets:
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet("/myServlet")
public class MyServlet extends HttpServlet {
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String input = request.getParameter("name");
System.out.println("You entered: " + input);
}
}
In this example, the servlet uses the getParameter()
method to retrieve the user input from an HTML form.
These are just a few examples of how you can allow users to enter data in a Java program. The choice of approach depends on your specific application requirements and constraints.
Java do while loop with user input example
I'm happy to provide a response in English!
Let's create an example of a do-while
loop in Java that uses user input. In this program, we'll ask the user for their name and age, and then keep asking until they enter valid information.
Here's the code:
import java.util.Scanner;
public class DoWhileLoopExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
String userName;
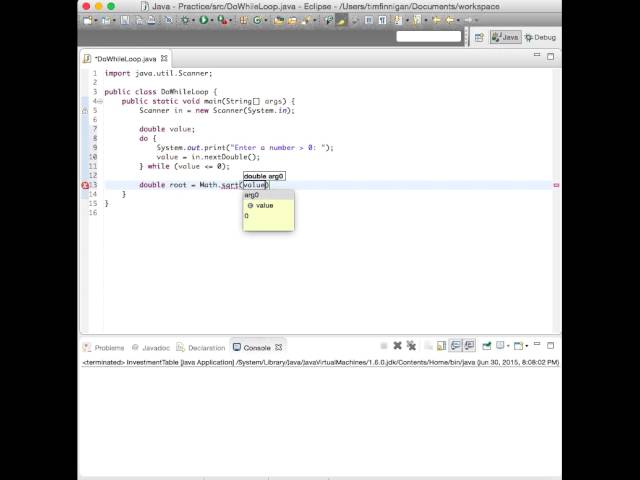
int userAge;
do {
System.out.print("Please enter your name: ");
userName = scanner.nextLine();
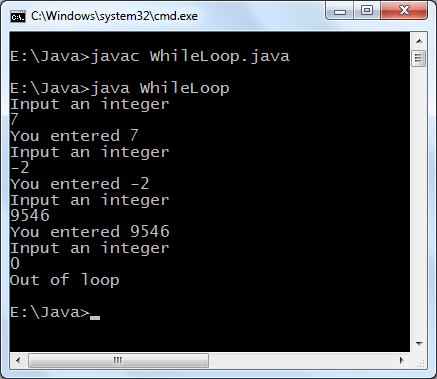
if (userName.length() < 2 || userName.length() > 20) {
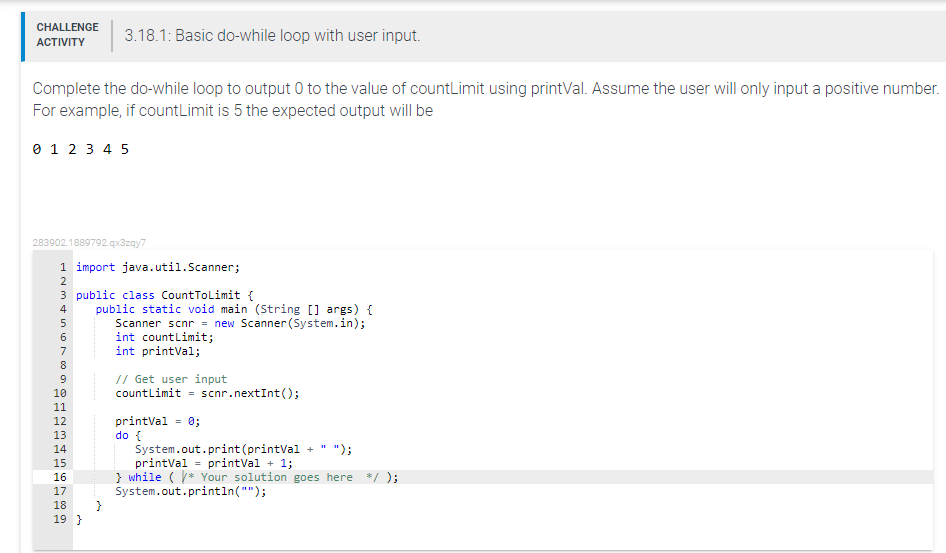
System.out.println("Your name should be between 2 and 20 characters.");
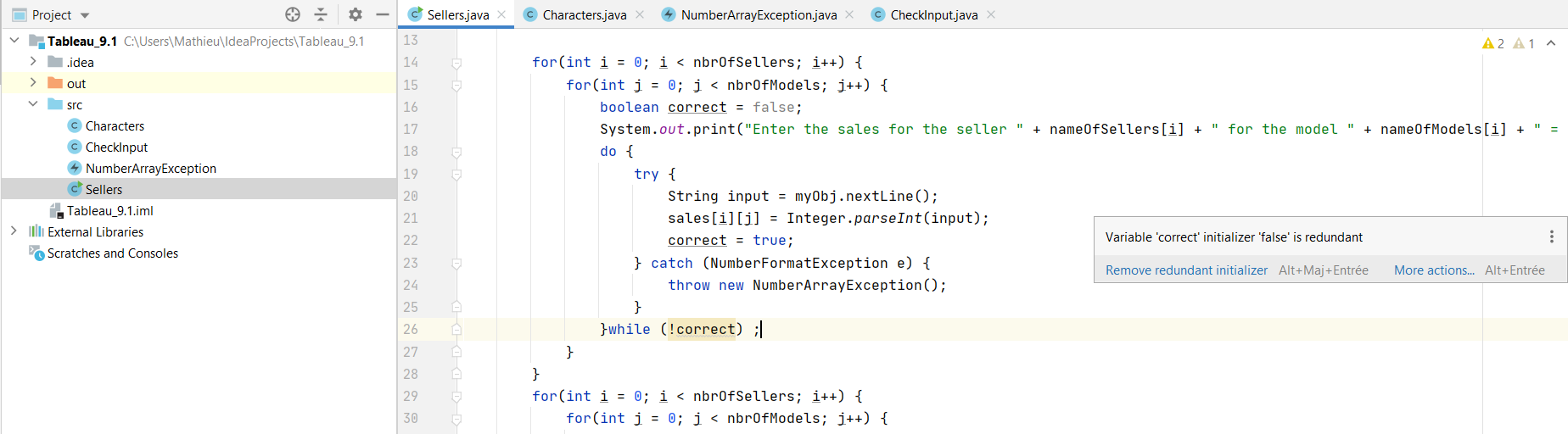
}
} while (userName.length() < 2 || userName.length() > 20);
do {
System.out.print("Please enter your age: ");
userAge = scanner.nextInt();
if (userAge < 1) {
System.out.println("Your age should be at least 1.");
}
} while (userAge < 1);
System.out.println("Welcome, " + userName + "! You are " + userAge + " years old.");
}
}
In this example, we use two do-while
loops. The first loop ensures that the user enters a valid name by checking its length (between 2 and 20 characters). The second loop does the same for the age (at least 1).
Here's how it works:
We import theScanner
class to read user input. We declare two variables: userName
and userAge
. In the first do-while
loop, we keep asking for the user's name until they enter a valid length (between 2 and 20 characters). If their entry is too short or too long, we print an error message. Once they enter a valid name, we move on to the second do-while
loop. In this loop, we keep asking for the user's age until it's at least 1. If they enter a non-positive number, we print another error message. Finally, after both loops complete successfully, we display a welcome message with their name and age.
When you run this program, you'll be prompted to enter your name and age. Keep entering invalid information (like a 3-letter name or an age of -1), and the program will keep asking until you provide valid input!
Feel free to modify the loops to suit your specific needs. Happy coding!