Which method releases the lock in Java?
Which method releases the lock in Java?
I'd be happy to explain which methods can release a lock in Java.
In Java, synchronization is used to achieve thread safety by using locks (monitors) on critical sections of code. A lock is essentially a binary flag that indicates whether a certain block of code is currently being executed by one thread or another.
When you acquire a lock, it means you are attempting to gain access to a shared resource (like a bank account). This is typically done using the synchronized
keyword. If two threads both attempt to acquire the same lock simultaneously, only one will succeed and the other will be forced to wait until the first thread releases the lock.
Now, there are several methods in Java that can release a lock:
unlock()
: This method is not directly available in the standard library, but you could create your own implementation using Object.notify()
or Thread.yield()
.
Example:
synchronized (lock) {
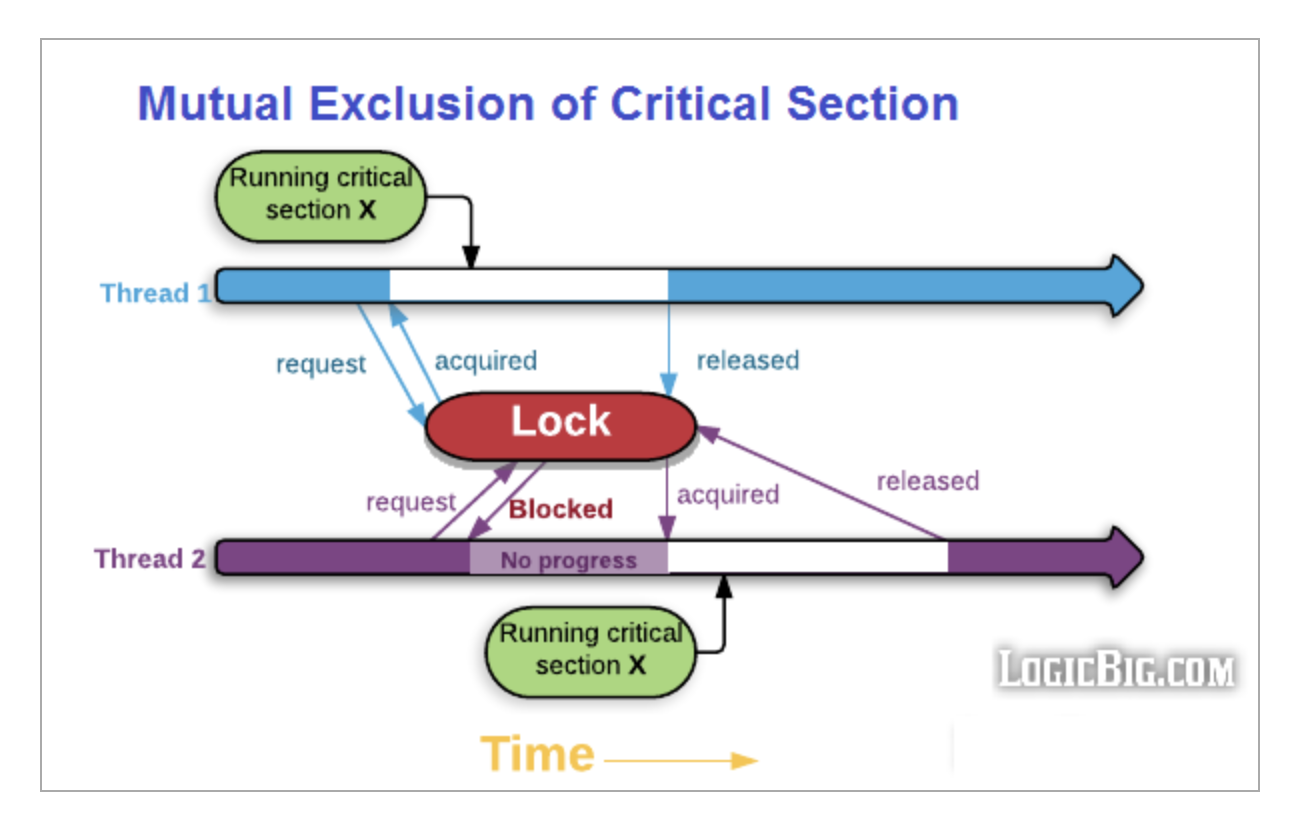
try {
// critical section
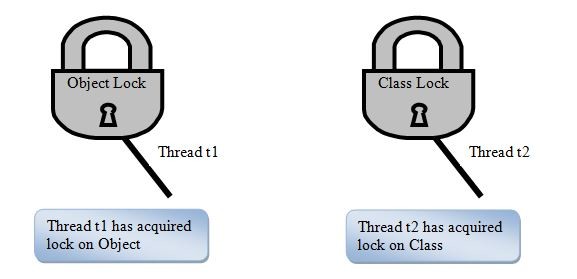
} finally {
lock.unlock(); // or notifyAll(), or yield()
}
}
release()
: This is a method from the ReentrantLock
class, which is available in the java.util.concurrent.locks
package.
Example:
final ReentrantLock lock = new ReentrantLock();
try {
lock.lock(); // Acquire
// critical section
} finally {
lock.unlock(); // Release
}
notify()
: This method wakes up one thread that is waiting on the same lock.
Example:
synchronized (lock) {
try {
// wait for some condition to be met
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
} finally {
lock.notify(); // Release and notify other threads
}
}
notifyAll()
: This method wakes up all the threads that are waiting on the same lock.
Example:
synchronized (lock) {
try {
// wait for some condition to be met
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
} finally {
lock.notifyAll(); // Release and notify other threads
}
}
In all these cases, releasing a lock means that the thread has finished with its critical section of code, allowing other threads to potentially acquire the same lock if they are waiting.
Remember, locks should always be released when their use is no longer required. Failing to do so can lead to deadlocks, livelocks, or even starvation, as other threads may never get a chance to access shared resources.
What is object lock and when is a thread required in Java?
I'd be happy to explain object locking and thread requirements in Java!
Object Locking (Synchronization)
In Java, object locking, also known as synchronization, refers to the process of controlling access to shared resources by multiple threads or processes concurrently executing the same program. In other words, it ensures that only one thread can modify a shared resource at any given time.
Java provides various mechanisms for achieving object locking:
Synchronized methods: Marking a method with thesynchronized
keyword guarantees that only one thread can execute this method at a time. Synchronized blocks: The synchronized
statement allows you to specify a block of code that should be executed in a thread-safe manner. Lock objects: You can create a lock object using the ReentrantLock
or Lock
class from the java.util.concurrent.locks
package.
Object locking is crucial in multi-threaded environments because it helps prevent:
Data corruption: Multiple threads modifying shared data concurrently, leading to inconsistent results. Inconsistent state: Threads interfering with each other's execution, causing unexpected behavior.Thread Requirements
A thread is required in Java when:
Performing I/O operations: When a program needs to perform input/output (I/O) operations, such as reading or writing files, network communication, or user input, a separate thread can handle these tasks without blocking the main execution thread. Long-running computations: Threads are useful for executing time-consuming computations that don't require constant attention from the main thread, allowing the program to continue processing other tasks in parallel. Real-time applications: In real-time systems where predictable and consistent responses are critical, threads help ensure that tasks are executed in a timely and controlled manner.Some scenarios where you might need multiple threads include:
Handling user input and GUI events concurrently Performing background tasks like data processing or updates while keeping the main thread responsive to user interactions Implementing parallel algorithms for scientific computing, simulations, or data analysisIn summary, object locking ensures thread-safe access to shared resources, preventing data corruption and inconsistent state. Threads are required in Java when you need to:
Perform I/O operations Execute long-running computations Implement real-time applications that require predictable and consistent responses.By understanding the concepts of object locking and thread requirements, you'll be better equipped to write robust, efficient, and scalable Java programs!