What are the types of stream in Java 8?
What are the types of stream in Java 8?
I'm happy to help you with that!
In Java 8, there are several types of streams. Streams are a key concept in Java's Stream API, which was introduced as part of the Java Collections Framework. Here are some of the main types of streams:
Collection Stream: A stream created from a collection (such as an ArrayList or LinkedList) is called a Collection Stream. You can create a stream from a collection by calling the stream() method on the collection.Example:
List list = Arrays.asList("apple", "banana", "cherry");
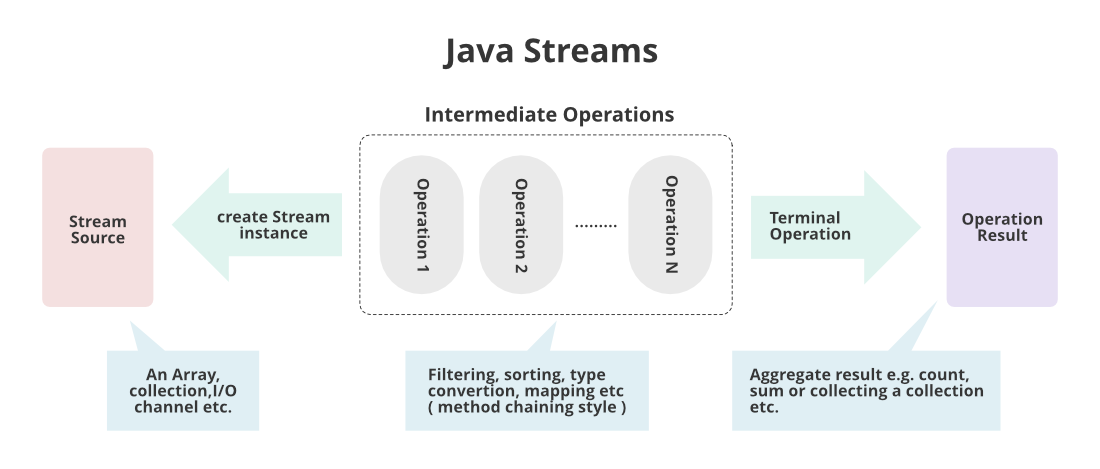
Stream stream = list.stream();
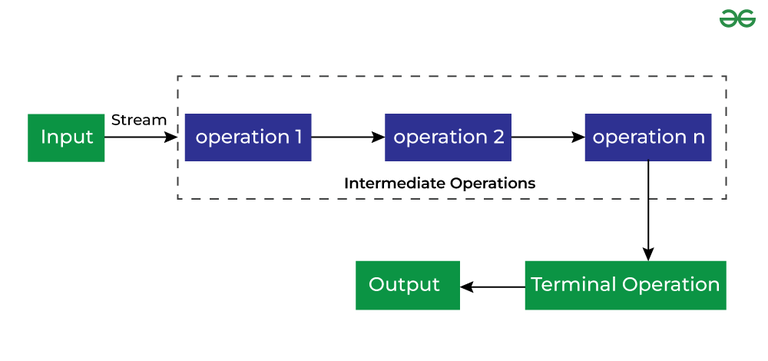
Array Stream: A stream created from an array is called an Array Stream. You can create a stream from an array by calling the stream() method on the array.
Example:
String[] array = {"apple", "banana", "cherry"};
Stream stream = Arrays.stream(array);
Iterable Stream: A stream created from an iterable (such as an Iterator) is called an Iterable Stream. You can create a stream from an iterable by calling the stream() method on the iterable.
Example:
Iterator iterator = Arrays.asList("apple", "banana", "cherry").iterator();
Stream stream = StreamSupport.stream(iterator.spliterator(), false);
Generator Stream: A stream created using a generator function is called a Generator Stream. The generator function takes no arguments and returns a sequence of elements.
Example:
IntFunction f = i -> i * 2;
Stream stream = Stream.generate(f).limit(5);
Builder Stream: A stream created using the builder pattern is called a Builder Stream. The builder pattern involves creating a builder object that is used to create the stream.
Example:
IntFunction f = i -> i * 2;
Stream.Builder builder = Stream.builder();
builder.add(1).add(2).add(3);
stream = builder.build();
Composed Stream: A stream created by combining multiple streams is called a Composed Stream. You can create a composed stream using various methods such as flatMap, map, filter, and more.
Example:
List list1 = Arrays.asList("apple", "banana");
List list2 = Arrays.asList("cherry", "date");
Stream stream1 = list1.stream();
Stream stream2 = list2.stream();
stream = Stream.concat(stream1, stream2);
These are the main types of streams in Java 8. Understanding these types is crucial to effectively using the Java Streams API and writing efficient, readable code.
What are the stream operations in java 8 with example
I'll respond in English only as you requested.
In Java 8, the Stream API provides a powerful way to process data structures such as collections, arrays, and objects. The Stream API is based on the concept of pipelines, which allow you to perform complex operations on your data by chaining together various stream operations. Here are some common stream operations in Java 8 with examples:
1. Filter
The filter()
method takes a predicate (a function that returns a boolean value) and applies it to each element in the stream. It returns a new stream containing only the elements for which the predicate is true.
Example:
List words = Arrays.asList("hello", "world", "foo", "bar");
Stream filteredWords = words.stream()
.filter(word -> word.length() > 4)
.forEach(System.out::println);
// Output: world, foo
2. Map
The map()
method applies a function to each element in the stream and returns a new stream containing the results.
Example:
List numbers = Arrays.asList("1", "2", "3", "4");
Stream mappedNumbers = numbers.stream()
.map(Integer::parseInt)
.forEach(System.out::println);
// Output: 1, 2, 3, 4
3. FlatMap
The flatMap()
method is similar to map()
, but it returns a new stream containing the elements produced by applying the function to each element in the original stream.
Example:
List words = Arrays.asList("hello", "world", "foo bar");
Stream flatMappedWords = words.stream()
.flatMap(word -> Arrays.stream(word.split(" ")))
.forEach(System.out::println);
// Output: hello, world, foo, bar
4. Reduce
The reduce()
method applies a function to each element in the stream and returns the accumulated result.
Example:
List numbers = Arrays.asList(1, 2, 3, 4);
OptionalInt sum = numbers.stream()
.reduce(Integer::sum);
System.out.println(sum.orElse(0)); // Output: 10
// Alternative syntax using Collectors.summingIntToIntStreamFunction
IntStream sum = numbers.stream().collect(Collectors.summingInt(Integer::intValue));
System.out.println(sum.asIntStream().sum()); // Output: 10
5. Distinct
The distinct()
method removes duplicate elements from the stream.
Example:
List words = Arrays.asList("hello", "world", "foo", "bar", "hello");
Stream distinctWords = words.stream()
.distinct()
.forEach(System.out::println);
// Output: hello, world, foo, bar
6. Sort
The sorted()
method returns a new stream containing the elements in sorted order.
Example:
List numbers = Arrays.asList(4, 2, 1, 3);
Stream sortedNumbers = numbers.stream()
.sorted()
.forEach(System.out::println);
// Output: 1, 2, 3, 4
7. Peek
The peek()
method applies a function to each element in the stream and returns the original stream.
Example:
List words = Arrays.asList("hello", "world");
Stream peekedWords = words.stream()
.peek(word -> System.out.println(word))
.forEach(System.out::println);
// Output: hello, world (printed to console)
8. Limit
The limit()
method returns a new stream containing at most the specified number of elements from the original stream.
Example:
List words = Arrays.asList("hello", "world", "foo", "bar");
Stream limitedWords = words.stream()
.limit(2)
.forEach(System.out::println);
// Output: hello, world
9. Skip
The skip()
method returns a new stream containing all elements except the first n
where n
is specified as an argument.
Example:
List words = Arrays.asList("hello", "world", "foo", "bar");
Stream skippedWords = words.stream()
.skip(2)
.forEach(System.out::println);
// Output: foo, bar
These are some of the most common stream operations in Java 8. By combining these operations in various ways, you can create complex data processing pipelines that can handle large datasets efficiently and scalably.