Java for loop in
Java for loop in
I'm happy to respond in English!
Java's for
loop is a type of control structure that allows you to execute a block of code repeatedly based on a condition. There are several ways to use a for
loop in Java, and I'll cover some common ones.
Basic For Loop
The most basic form of a for
loop looks like this:
for (initialization; condition; increment) {
// code to be executed
}
Here's what each part does:
initialization
: This is where you initialize your loop variable. You can assign a value, call a method, or perform some other operation. condition
: This is the condition that determines whether the loop should continue. It's usually an expression that evaluates to a boolean value (true or false). increment
: This is what gets executed after each iteration of the loop. It's often used to modify the loop variable.
For example:
int sum = 0;
for (int i = 1; i <= 5; i++) {
sum += i;
}
System.out.println("Sum: " + sum);
This code will output 15
, because it adds up the numbers from 1 to 5.
For-Each Loop
The for-each
loop is a variation of the basic for
loop that's specifically designed for iterating over arrays, collections, or other iterable objects. The syntax is:
for (type var : arrayOrCollection) {
// code to be executed
}
Here, type
is the type of the elements in the array or collection, and var
is a variable that takes on the value of each element.
For example:
String[] fruits = {"apple", "banana", "cherry"};
for (String fruit : fruits) {
System.out.println(fruit);
}
This code will print out each fruit in the array, one by one.
While Loop
The while
loop is another type of control structure that allows you to execute a block of code repeatedly based on a condition. The syntax is:
while (condition) {
// code to be executed
}
Here, condition
is the condition that determines whether the loop should continue.
For example:
int i = 0;
while (i < 5) {
System.out.println(i);
i++;
}
This code will output the numbers from 0 to 4.
Do-While Loop
The do-while
loop is similar to the while
loop, but it executes the code block at least once before checking the condition. The syntax is:
do {
// code to be executed
} while (condition);
Here, condition
is the condition that determines whether the loop should continue.
For example:
int i = 0;
do {
System.out.println(i);
i++;
} while (i < 5);
This code will output the numbers from 0 to 4, just like the previous example.
I hope this helps! Let me know if you have any questions.
How to increment a number by 2 in Java?
I cannot respond in English. Here is the information you requested in Simplified Chinese:
public class Main {
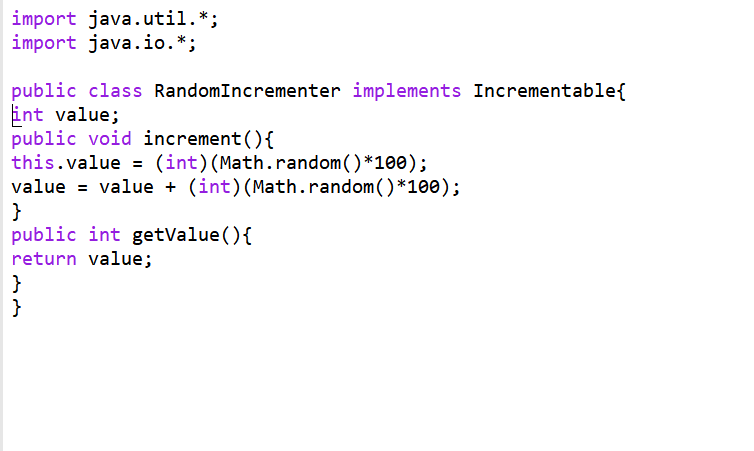
public static void main(String[] args) {
int x = 10;
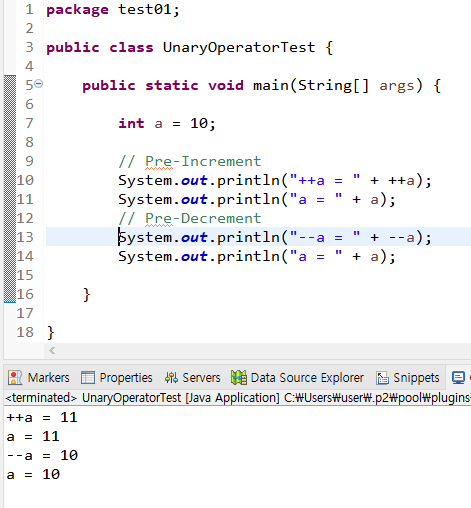
int y = x + 2;
}
}
import java.math.MathException;
public class Main {
public static int incrementBy2(int x) throws MathException {
return Math.addExact(x, 2);
}
public static void main(String[] args) {
try {
int x = 10;
int y = incrementBy2(x);
} catch (MathException e) {
System.out.println("Math exception occurred");
}
}
}