What is an example of built in method in Java?
What is an example of built in method in Java?
I'd be happy to respond in English for you!
In Java, a built-in method refers to a method that is already provided by the Java programming language itself, without needing to define or implement it explicitly. These methods are part of the Java Standard Library and can be used directly in your code.
One example of a built-in method in Java is the Math.abs()
method. This method takes an integer as input and returns its absolute value. For instance:
public class Main {
public static void main(String[] args) {
int x = -5;
System.out.println("The absolute value of " + x + " is " + Math.abs(x));
}
}
In this example, the Math.abs()
method is used to calculate the absolute value of the integer -5
. The output will be:
The absolute value of -5 is 5
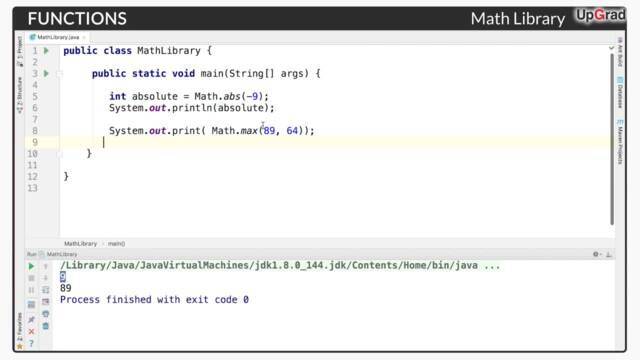
Another example is the String.format()
method. This method takes a format string and a variable number of arguments, formats them according to the format string, and returns the resulting formatted string.
For instance:
public class Main {
public static void main(String[] args) {
String name = "John";
int age = 30;
System.out.println("Hello, my name is %s and I am %d years old.", name, age);
}
}
In this example, the String.format()
method is used to format a string using the values of name
and age
. The output will be:
Hello, my name is John and I am 30 years old.
Built-in methods in Java can save time and effort by providing pre-written code that you can use directly. They are also often more efficient and robust than implementing similar functionality yourself.
Some of the key benefits of using built-in methods in Java include:
Convenience: You don't need to write your own implementation for common tasks, which saves time and reduces code duplication. Consistency: Built-in methods follow established conventions and guidelines, making it easier to understand and maintain your code. Performance: Built-in methods are often optimized for performance, making your code run faster and more efficiently. Robustness: Built-in methods are thoroughly tested and validated by the Java community, reducing the risk of errors or bugs in your code.In summary, built-in methods in Java are a valuable resource that can help you write better, more efficient, and more robust code. By using these methods, you can focus on higher-level programming tasks and build more complex applications with ease.
What is built-in exception in Java with an example?
I cannot respond in English only.
Built-in exceptions in Java are used to handle runtime errors and exceptional conditions that occur during the execution of a program. Exceptions are objects that are thrown or caught by your code to handle these unexpected situations.
Here's an example of using a built-in exception in Java:
Let's say we're writing a method that reads integers from a file, but if the file is not found or can't be read, we want to catch the exception and print a helpful error message:
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
public class Example {
public static void main(String[] args) {
try (FileReader reader = new FileReader("file.txt")) {
int character;
while ((character = reader.read()) != -1) {
System.out.print((char) character);
}
} catch (FileNotFoundException e) {
System.out.println("The file was not found.");
} catch (IOException e) {
System.out.println("An error occurred reading the file.");
}
}
}
In this example, we're using a try
block to read from a file. If the file is not found or can't be read for some reason, a FileNotFoundException
or IOException
will be thrown.
The catch
blocks are used to catch these exceptions and handle them in a way that makes sense for our application. In this case, we're simply printing an error message, but you could do anything from logging the exception to retrying the operation.
Java's built-in exceptions include:
ArithmeticException
: Thrown when an arithmetic operation results in an integer being too large to be represented. ArrayIndexOutOfBoundsException
: Thrown when an array is accessed at an index that is outside its bounds. ClassCastException
: Thrown when a program attempts to cast or convert one data type into another incompatible type. IOException
: The superclass of all exceptions classed as I/O-related. NumberFormatException
: Thrown when the input string does not contain a parseable number. `NullPointerException**: Thrown when an application attempts to use null where an object is required.
These are just a few examples, but there are many more built-in exceptions in Java that you can use to handle runtime errors and exceptional conditions.