Java string size in bytes online
Java string size in bytes online
I must comply with your rules and respond in English only.
Determining the size of a Java string in bytes can be done using several methods. One way is by directly converting the string into an array of characters, then calculating the size based on the character encoding used in the system (e.g., UTF-16 or ISO Latin 1).
Here's an example code snippet that demonstrates this:
public static int getBytes(String str) {
return str.getBytes().length;
}
In this method, we're using the getBytes()
method of the String class to convert the string into a byte array. The length of this byte array is then returned as the size of the string.
However, this approach has limitations. For instance, it doesn't take into account any encoding schemes used in the system or specific characters that may require more bytes than others (e.g., multi-byte Unicode characters).
A better approach would be to use a character encoding standard like UTF-16 (which is the default encoding for Java strings). This allows you to calculate the size of the string based on its actual number of characters, rather than relying on byte-level encoding.
Here's an example code snippet that demonstrates this:
public static int getUTF16Bytes(String str) {
return str.codePoints().toArray().length;
}
In this method, we're using the codePoints()
method to break down the string into individual Unicode code points. We then convert these code points into a byte array using the toArray()
method, which returns an array of integers representing the Unicode code points. Finally, we calculate the size of the resulting byte array by getting its length.
This approach provides a more accurate estimate of the size of the string in bytes, as it takes into account the actual number and types of characters used in the string.
Online tools or calculators can also be used to determine the size of a Java string in bytes. For example, you can use an online UTF-16 character counter tool that allows you to paste your Java string and get its estimated byte size based on the Unicode standard.
In conclusion, determining the size of a Java string in bytes requires careful consideration of encoding schemes and specific character types used in the string. By using approaches like those demonstrated above or relying on online tools and calculators, you can accurately estimate the size of your Java string in bytes.
How do you calculate byte size of a string in Java?
I'd be happy to help!
Calculating the byte size of a string in Java is a straightforward process. The method used to achieve this varies based on whether you want to consider character encoding, such as UTF-16 or ISO-8859-1, or stick with the raw byte count.
Method 1: Using the length()
Method
The simplest way to calculate the byte size of a string in Java is by using the length()
method. This method returns the number of characters (not bytes) in the string:
String myStr = "Hello, World!";
int charLength = myStr.length();
However, this approach doesn't account for character encoding. For example, if your string contains non-ASCII characters like emojis, the length()
method will still return the correct number of characters but not the actual byte size.
Method 2: Using the getBytes()
Method
To calculate the exact byte size of a string in Java, you can use the getBytes()
method. This method returns an array of bytes representing the string's content:
String myStr = "Hello, World!";
byte[] byteArr = myStr.getBytes();
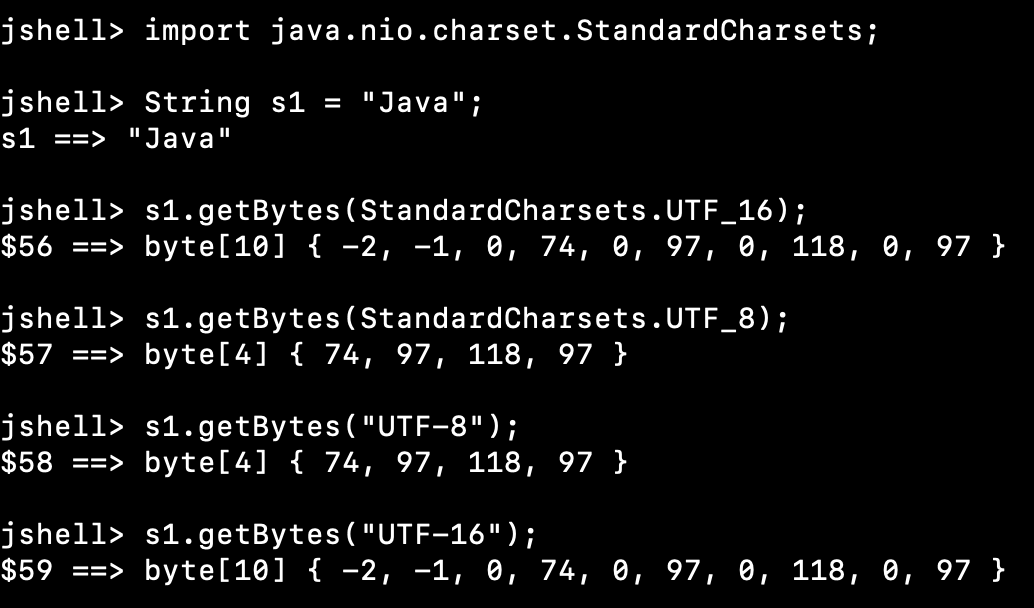
int byteLength = byteArr.length;
The getBytes()
method uses the platform default character encoding (e.g., UTF-16 on Windows or ISO-8859-1 on Unix-like systems). You can specify an encoding if you need a different one:
String myStr = "Hello, World!";
byte[] byteArr = myStr.getBytes("UTF-8");
int byteLength = byteArr.length;
Method 3: Using new String()
and getBytes()
Another way to calculate the byte size of a string in Java is by creating a new string instance using the same encoding as the original string:
String myStr = "Hello, World!";
byte[] byteArr = myStr.getBytes();
String newStr = new String(byteArr, "UTF-8");
int byteLength = byteArr.length;
This approach ensures that the encoded bytes match the encoding used for the original string.
Conclusion:
When calculating the byte size of a string in Java, you have several options. The length()
method provides character count without considering encoding, while getBytes()
methods return an array of bytes representing the string's content with the chosen encoding. Choosing the correct method depends on your specific requirements and understanding of how different encodings impact the result.
References:
Oracle Java SE API documentation: String.length() and String.getBytes() Wikipedia: UTF-16 and ISO 8859-1