How to check the data type Java?
How to check the data type Java?
I'd be happy to help you with that!
In Java, you can check the data type of a variable using various methods. Here are some ways to do it:
Using theinstanceof
Operator: You can use the instanceof
operator to check if an object is an instance of a specific class or interface. This method works for reference types only.
Object obj = new String("Hello");
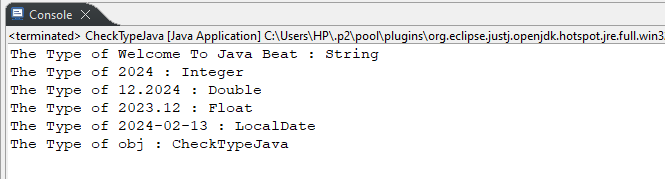
boolean isString = obj instanceof String; // returns true
Using the .getClass()
Method: You can use the getClass()
method to get the runtime class of an object and then compare it with a specific class or interface.
Object obj = new String("Hello");
Class<?> clazz = obj.getClass();
boolean isString = String.class.isAssignableFrom(clazz); // returns true
Using Reflection: You can use Java reflection to get the declared type of a variable using the getDeclaringClass()
method.
Object obj = new String("Hello");
Class<?> clazz = obj.getClass().getDeclaredClasses()[0];
boolean isString = String.class.equals(clazz); // returns true
Using an Enum: You can use a custom enum to represent different data types and then use the equals()
method to compare the actual type of an object with the expected type.
enum DataType {
INTEGER, FLOAT, STRING
}
Object obj = "Hello";
DataType dataType = getDataType(obj); // returns STRING
Here's a sample implementation of the above approach:
public class Main {
public static void main(String[] args) {
Object obj1 = 123; // int value
Object obj2 = 3.14f; // float value
Object obj3 = "Hello"; // string value
System.out.println(getDataType(obj1)); // prints INTEGER
System.out.println(getDataType(obj2)); // prints FLOAT
System.out.println(getDataType(obj3)); // prints STRING
}
public static DataType getDataType(Object obj) {
if (obj instanceof Integer) {
return DataType.INTEGER;
} else if (obj instanceof Float) {
return DataType.FLOAT;
} else if (obj instanceof String) {
return DataType.STRING;
} else {
return null; // unknown type
}
}
public enum DataType {
INTEGER, FLOAT, STRING
}
}
These are some of the ways you can check the data type of a variable in Java. The approach you choose depends on your specific requirements and constraints.
How to check if type is primitive Java?
To determine whether a given Java type is primitive or not, you can follow these steps:
Use theisPrimitive()
method: You can use the isPrimitive()
method provided by the Java Type system. This method belongs to the java.lang.reflect.Type
class and returns a boolean value indicating whether the specified Type
object represents a primitive type.
Here's an example:
import java.lang.reflect.Type;
public class Main {
public static void main(String[] args) {
// Create a Type object for an int primitive type
Type intType = int.class.asSubtype(Type.class);
if (intType.isPrimitive()) {
System.out.println("The type is primitive");
} else {
System.out.println("The type is not primitive");
}
}
}
Check the getTypeName()
method: You can also use the getTypeName()
method, which belongs to the same java.lang.reflect.Type
class. This method returns a string that represents the name of the type.
For example:
import java.lang.reflect.Type;
public class Main {
public static void main(String[] args) {
// Create a Type object for an int primitive type
Type intType = int.class.asSubtype(Type.class);
String typeName = intType.getTypeName();
if (typeName.equals("int") || typeName.equals("boolean")) {
System.out.println("The type is primitive");
} else {
System.out.println("The type is not primitive");
}
}
}
In both cases, the int
type will be recognized as a primitive type because its getTypeName()
method returns the string "int", which matches one of the expected values.
Key differences between primitives and non-primitives:
Primitive types are fundamental data types that are built into the Java programming language. Examples include int, boolean, char, byte, short, long, float, and double. Non-primitive types, on the other hand, are classes or interfaces created by developers to represent more complex data structures or abstract concepts.Benefits of checking for primitive types:
Improved code readability: By explicitly checking whether a type is primitive or not, you can make your code easier to understand and maintain. Enhanced error handling: When working with non-primitive types, it's essential to handle null references and other exceptions carefully. Checking the type of a variable helps you detect potential errors earlier in your coding process.Real-world scenarios:
Data validation and sanitization: In web applications, you might need to validate user input data. By checking whether a submitted value is a primitive type (e.g., integer or boolean), you can ensure that the data conforms to expected formats. Dynamic data processing: When working with large datasets or performing complex calculations, it's crucial to differentiate between primitive and non-primitive types. This helps you optimize your code for better performance.In conclusion, checking whether a Java type is primitive or not is an essential step in developing robust and efficient software applications. By using the isPrimitive()
method or the getTypeName()
method, you can gain valuable insights into the nature of your data and make informed decisions about how to handle it effectively.