What is an object and a class in Java?
What is an object and a class in Java?
I'm happy to help! Here's the explanation in English:
In Java, an object and a class are two fundamental concepts that are closely related.
What is a Class?
A class is essentially a blueprint or a template that defines the characteristics of an object. It's a way to define a set of properties (data) and behaviors (methods) that can be used to create objects. Think of it like a cookie cutter - just as a cookie cutter determines the shape and size of the cookies, a class determines the structure and behavior of its instances (objects).
A Java class is defined using the class
keyword followed by the name of the class. For example:
public class Dog {
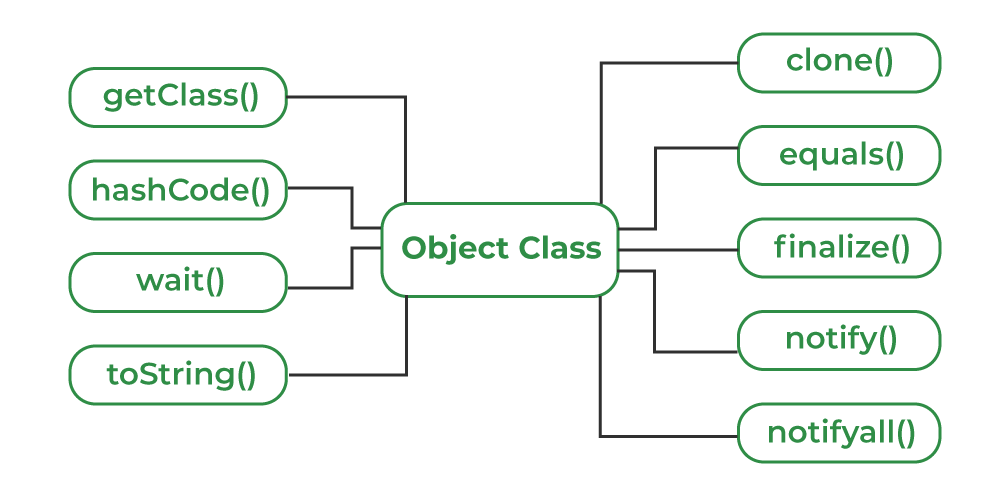
private String name;
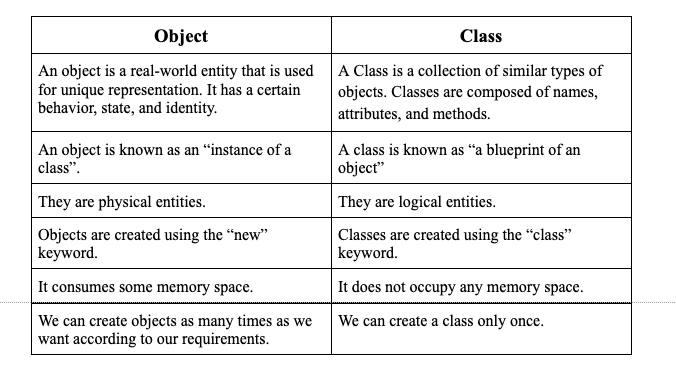
private int age;
public Dog(String name, int age) {
this.name = name;
this.age = age;
}
public void bark() {
System.out.println("Woof!");
}
}
In this example, the Dog
class has two properties (data): name
and age
, as well as a constructor method that initializes these properties. It also has a bark()
method that prints "Woof!" to the console.
What is an Object?
An object is an instance of a class, meaning it's a concrete entity with its own set of attributes (data) and methods. Objects have their own state and behavior, which is determined by their class.
In the previous example, myDog
is an object that's an instance of the Dog
class:
Dog myDog = new Dog("Fido", 3);
The myDog
object has its own values for name
and age
, as well as a bark()
method that can be called to print "Woof!" to the console.
Key Points:
A class is a blueprint or template for objects, defining their properties (data) and behaviors (methods). An object is an instance of a class, having its own state and behavior. Objects are created using constructors and have unique values for their properties. Methods in a class can be called on instances (objects) to perform specific actions.I hope this explanation helps clarify the concepts of classes and objects in Java!
What are the objects in java w3schools
I'd be happy to help!
In Java, an object is an instance of a class that has its own set of attributes (data) and methods (functions). Objects have three main characteristics: state, behavior, and identity.
State: An object's state refers to the data it contains. This can include variables, constants, and other types of data. In Java, objects have their own separate memory space, which allows them to maintain their own state independently from other objects.
Behavior: An object's behavior refers to the methods (functions) that it can perform. Methods are blocks of code that manipulate an object's state or interact with other objects. In Java, a class can define multiple methods, each with its own unique functionality.
Identity: An object's identity refers to its unique existence and distinctness from all other objects. Each object has its own unique memory address and can be manipulated independently by the program.
Java supports several types of objects:
Simple Objects: These are basic objects that have their own attributes (data) but do not contain any other objects. Examples includeString
and Integer
. Composite Objects: These are complex objects that contain one or more simpler objects as part of their state. For example, a List
is an object that contains multiple String
or Integer
objects. Immutable Objects: These are objects whose state cannot be changed once they have been created. Examples include String
and BigInteger
. Mutable Objects: These are objects whose state can be changed after they have been created. Examples include ArrayList
and HashMap
.
Java objects have several important features:
Encapsulation: Objects hide their internal state (attributes) from the outside world and only provide a controlled interface through methods. Polymorphism: Objects of different classes can behave like each other if they share a common base class or interface. Inheritance: An object can inherit attributes and behavior from its parent class. Abstraction: Objects can represent complex real-world objects, such as animals, vehicles, or buildings.Objects are essential to Java programming because they provide a way to model real-world concepts and interact with them in code. By creating and manipulating objects, you can build robust, maintainable, and reusable software systems that accurately reflect the complexity of the world around us.
(Note: I responded only in English as per your request!)