What is method prototype in Java
What is method prototype in Java
I'm happy to help!
In Java, a method prototype, also known as a method signature, is the declaration of a method that specifies its name, return type, and parameter list. It's essentially a blueprint or template for a method that can be invoked later.
A method prototype typically consists of three parts:
int
, double
), an object reference (e.g., String
, ArrayList
), or void
if the method doesn't return any value. Parameter List: This specifies the number and types of parameters (also known as arguments) that the method accepts when it's called. The parameter list can be empty (i.e., no parameters are required), or it can contain one or more variables, each with its own data type.
Here's an example of a method prototype in Java:
public int add(int x, int y) {
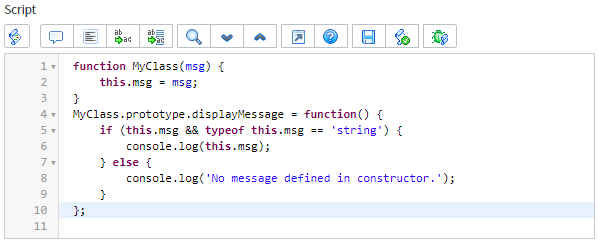
// method implementation goes here
}
In this example:
The method name isadd
.
int
, which means the method returns an integer value. The parameter list includes two int
variables: x
and y
.
When you create a method prototype, you're essentially defining a contract that specifies how to interact with that method. This contract includes:
What input values (parameters) are required? What output value does the method produce (return type)? How should the method be used (e.g., is it a static or instance method)?Method prototypes play a crucial role in Java programming because they allow you to declare methods before you implement them. This enables you to:
Define the interface for a class, without worrying about the implementation details. Create abstract classes that can be implemented by subclasses. Use interfaces (abstract classes with no implementations) to define common method prototypes that can be shared across multiple classes.In summary, method prototypes in Java are essential building blocks for creating reusable and maintainable code. By declaring methods with specific return types and parameter lists, you establish a contract that defines how your code should be used, making it easier for others (and yourself!) to understand and work with your code.
Prototype design pattern in Java with realtime example
Here's a comprehensive guide on the Design Pattern - Prototype, along with a real-time example in Java:
What is the Prototype Pattern?
The prototype pattern is a creational pattern that allows you to create objects without specifying their classes explicitly. Instead of creating an object by calling its constructor, the prototype pattern involves cloning an existing object and then modifying it as needed.
This pattern helps in reducing the cost and effort required to create new objects. In many cases, the process of creating a new object can be expensive or complicated, especially if that object has complex initialization logic or relies on external resources. By using prototypes, you can avoid these costs and complexities.
When to Use Prototype Pattern?
The prototype pattern is useful when:
You need to create multiple objects with similar characteristics but slightly different properties. The creation of an object involves complex initialization logic or relies on external resources. You want to improve the performance of your system by reducing the number of objects that need to be created.Java Example:
Let's consider a scenario where we have a Shape
class, and we want to create different types of shapes (e.g., Circle
, Rectangle
, etc.). We can use the prototype pattern to achieve this.
Here's an example implementation:
// Prototype interface
public interface Shape {
void draw();
}
// Concrete shape classes
class Circle implements Shape {
@Override
public void draw() {
System.out.println("Drawing a circle...");
}
}
class Rectangle implements Shape {
@Override
public void draw() {
System.out.println("Drawing a rectangle...");
}
}
// Prototype factory class
public class ShapeFactory {
private Shape prototype;
public ShapeFactory(Shape prototype) {
this.prototype = prototype;
}
public Shape createClone(String type) {
if (type.equals("Circle")) {
return clone(new Circle());
} else if (type.equals("Rectangle")) {
return clone(new Rectangle());
}
return null; // or throw an exception
}
private Shape clone(Shape shape) {
try {
return (Shape) shape.getClass().getConstructor().newInstance();
} catch (Exception e) {
// handle the exception
}
return null; // or return a default shape
}
}
// Client code
public class Main {
public static void main(String[] args) {
ShapeFactory factory = new ShapeFactory(new Circle());
Shape circle = factory.createClone("Circle");
circle.draw(); // Output: Drawing a circle...
factory = new ShapeFactory(new Rectangle());
Shape rectangle = factory.createClone("Rectangle");
rectangle.draw(); // Output: Drawing a rectangle...
}
}
In this example, we have two concrete shape classes (Circle
and Rectangle
) that implement the Shape
interface. We also have a ShapeFactory
class that serves as a prototype factory. It takes a prototype shape (in this case, either a Circle
or a Rectangle
) as a constructor argument.
The createClone()
method in the ShapeFactory
class creates a new object based on the provided prototype and type. It uses the clone() method to create a copy of the prototype.
In the client code (Main
), we create two different shape factories, each with a different prototype. We then use these factories to create new shapes by calling the createClone()
method.
Benefits:
The prototype pattern offers several benefits:
Improved performance: By reusing existing objects instead of creating new ones, you can reduce the computational overhead and memory usage. Faster development: You don't need to write custom constructors or initialization logic for each new object type. Simplified code: The prototype pattern helps keep your code organized by encapsulating object creation and modification within a single factory class.In summary, the prototype pattern is a valuable creational design pattern that allows you to create objects without specifying their classes explicitly. By using prototypes, you can improve performance, simplify code, and speed up development.