W3schools java loops list
W3schools java loops list
I'd be happy to help!
Java Loops: A Comprehensive Guide
In Java, loops are used to execute a block of code repeatedly for a specified number of iterations. There are three primary types of loops in Java:
For Loop: Thefor
loop is used to iterate over an array, collection, or range of values.
Example:
int[] scores = {90, 80, 70, 60};
for (int score : scores) {
System.out.println(score);
}
This code will print each score in the scores
array on a new line.
while
loop is used to execute a block of code as long as a certain condition is true.
Example:
int i = 0;
while (i < 5) {
System.out.println(i);
i++;
}
This code will print the numbers 0, 1, 2, 3, and 4 on separate lines.
Do-While Loop: Thedo-while
loop is similar to the while
loop, but it checks the condition after executing the code block.
Example:
int i = 0;
do {
System.out.println(i);
i++;
} while (i < 5);
This code will also print the numbers 0, 1, 2, 3, and 4 on separate lines.
In addition to these primary types of loops, Java also provides:
Enhanced For Loop: Theenhanced for loop
is a type of for
loop that allows you to iterate over arrays, collections, or maps.
Labelled Loop: The labelled loop allows you to break out of a specific loop using theList colors = Arrays.asList("red", "green", "blue");
for (String color : colors) {
System.out.println(color);
}
break
statement.
Example:
outer: for (int i = 0; i < 2; i++) {
for (int j = 0; j < 2; j++) {
System.out.println(i + " " + j);
if (i == 1) break outer;
}
}
This code will print the numbers 0 0, 0 1, and then exit the loop.
Common Loop Control Statements
Java provides several loop control statements to manage the execution of loops:
break: Exits the current loop.for (int i = 0; i < 5; i++) {
if (i == 3) break;
System.out.println(i);
}
This code will print the numbers 0, 1, and 2, then exit the loop.
continue: Skips to the next iteration of the current loop.for (int i = 0; i < 5; i++) {
if (i == 3) continue;
System.out.println(i);
}
This code will print the numbers 0, 1, and 2, then skip to the next iteration.
return: Exits the current method.public static void main(String[] args) {
for (int i = 0; i < 5; i++) {
if (i == 3) return;
System.out.println(i);
}
}
This code will print the numbers 0, 1, and 2, then exit the main
method.
In conclusion, Java loops are an essential part of programming in Java. Understanding the different types of loops, as well as the loop control statements, is crucial for writing efficient and effective code.
W3schools java loops examples
Here are some examples of Java loops from W3Schools:
For Loop
A for loop iterates over a sequence (such as an array or a list) and executes a block of code once for each item in the sequence.
Example:
for (int i = 0; i < 5; i++) {
System.out.println("Hello, world!");
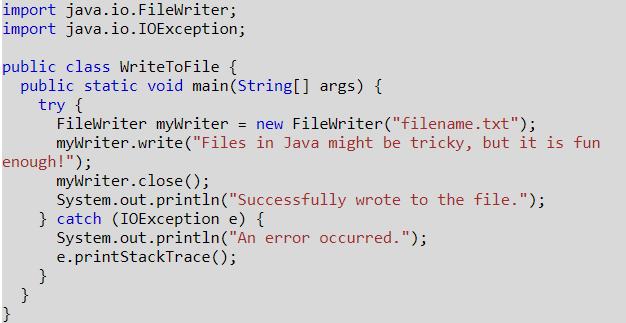
}
Output:
Hello, world!
Hello, world!
Hello, world!
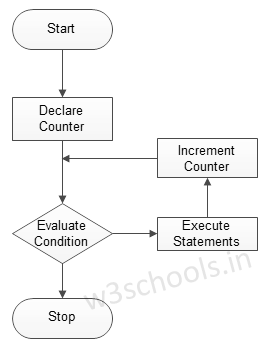
Hello, world!
Hello, world!
While Loop
A while loop executes a block of code as long as a specified condition is true.
Example:
int i = 0;
while (i < 5) {
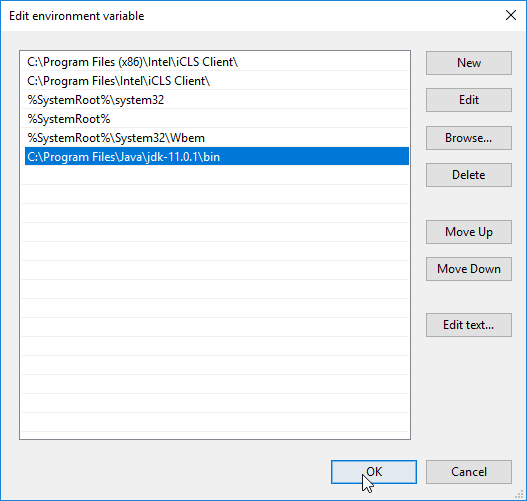
System.out.println("Hello, world!");
i++;
}
Output:
Hello, world!
Hello, world!
Hello, world!
Hello, world!
Hello, world!
Do-While Loop
A do-while loop is similar to a while loop, but it executes the block of code at least once before checking the condition.
Example:
int i = 0;
do {
System.out.println("Hello, world!");
i++;
} while (i < 5);
Output:
Hello, world!
Hello, world!
Hello, world!
Hello, world!
Hello, world!
Foreach Loop
A foreach loop iterates over a collection (such as an array or a list) and executes a block of code once for each item in the collection.
Example:
String[] fruits = {"Apple", "Banana", "Cherry"};
for (String fruit : fruits) {
System.out.println(fruit);
}
Output:
Apple
Banana
Cherry
Breaking Out of a Loop
Sometimes, you may want to exit a loop early. In Java, you can use the break
statement to do so.
Example:
for (int i = 0; i < 5; i++) {
if (i == 2) {
break;
}
System.out.println(i);
}
Output:
0
1
Loop Labels
You can use a loop label to identify which loop you are referring to when using the break
or continue
statements.
Example:
outer: for (int i = 0; i < 5; i++) {
inner: for (int j = 0; j < 5; j++) {
if (i == 2) {
break outer;
}
System.out.println(i + " " + j);
}
}
Output:
0 0
0 1
0 2
1 0
1 1
These are just a few examples of Java loops from W3Schools. I hope this helps! Let me know if you have any questions or need further clarification.