How to create your own string class in Java?
How to create your own string class in Java?
Creating a custom String class in Java allows you to define new methods and behavior for manipulating strings. Here's an example implementation:
First, let's start by defining the basic structure of the String class:
public class MyString {
private char[] chars;
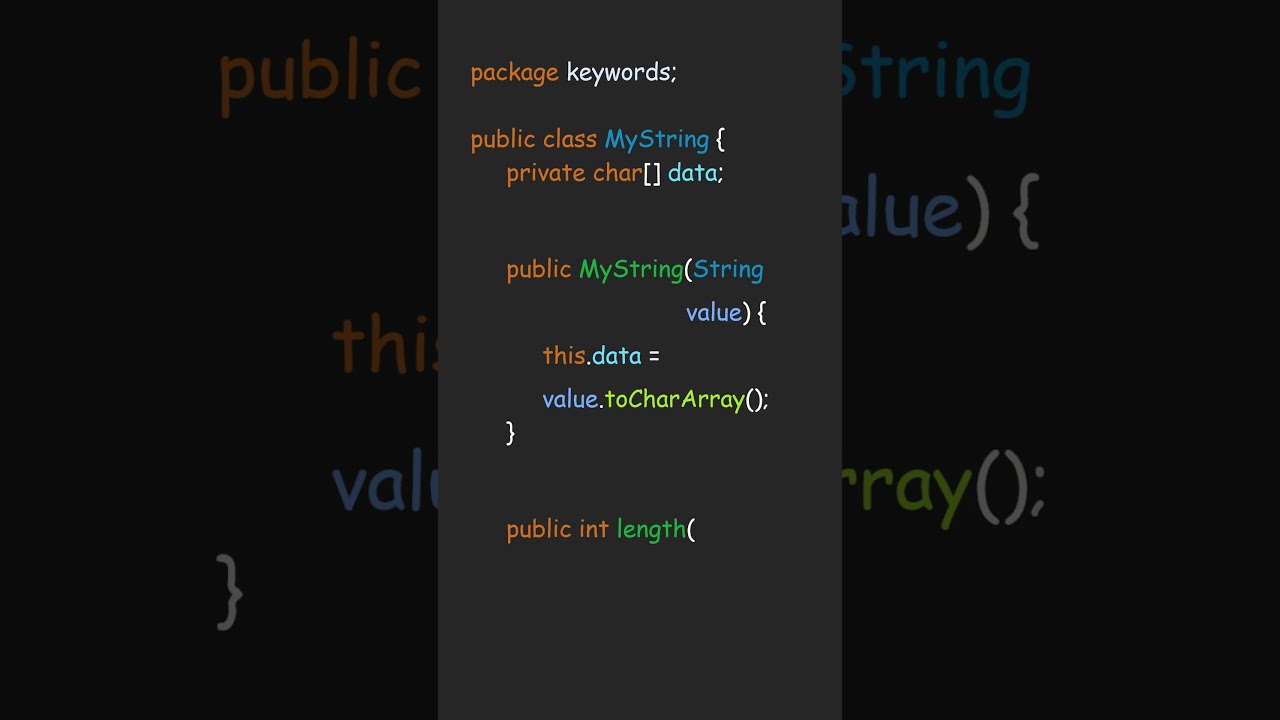
private int length;
public MyString(char[] chars) {
this.chars = chars;
this.length = chars.length;
}
}
In this implementation, we're using an array of characters (char[]
) to store the string data. The length
field keeps track of the number of characters in the string.
Next, let's implement some basic methods for manipulating the string:
public MyString concat(MyString other) {
char[] newChars = new char[length + other.length];
System.arraycopy(chars, 0, newChars, 0, length);
System.arraycopy(other.chars, 0, newChars, length, other.length);
return new MyString(newChars);
}
public boolean contains(MyString substring) {
for (int i = 0; i <= length - substring.length; i++) {
boolean match = true;
for (int j = 0; j < substring.length; j++) {
if (chars[i + j] != substring.chars[j]) {
match = false;
break;
}
}
if (match) return true;
}
return false;
}
public MyString substring(int start, int end) {
char[] newChars = new char[end - start];
System.arraycopy(chars, start, newChars, 0, end - start);
return new MyString(newChars);
}
The concat
method concatenates two strings by creating a new array that combines the characters of both strings. The contains
method checks if a given substring is present in the string by iterating through the characters and comparing them with the characters in the substring. The substring
method extracts a portion of the string, starting from the start
index up to but not including the end
index.
You can also override the toString
method to provide a human-readable representation of your string:
public String toString() {
StringBuilder sb = new StringBuilder();
for (char c : chars) {
sb.append(c);
}
return sb.toString();
}
Now, let's test our custom String class with some example code:
public static void main(String[] args) {
MyString s1 = new MyString(new char[] {'H', 'e', 'l', 'l', 'o' });
MyString s2 = new MyString(new char[] {'W', 'o', 'r', 'l', 'd'});
System.out.println(s1.toString()); // Output: Hello
System.out.println(s2.toString()); // Output: World
MyString concatResult = s1.concat(s2);
System.out.println(concatResult.toString()); // Output: HelloWorld
boolean containsResult = s1.contains(new MyString(new char[] {'e', 'l' }));
System.out.println(containsResult); // Output: true
MyString substringResult = s1.substring(1, 3);
System.out.println(substringResult.toString()); // Output: el
}
This example demonstrates basic usage of the custom String class. You can add more methods and behavior as needed to fit your specific use case.
Note that this is a simplified implementation and you may want to consider adding additional features such as handling null or empty strings, implementing equals
and hashCode
, and optimizing performance for large strings.
String class in java with example
I'd be happy to help!
The String
class in Java is a fundamental part of the language, representing a sequence of characters. Here's an overview and some examples:
What is a String?
In Java, a String
is an object that represents a sequence of characters. It's a immutable class, meaning once created, its value cannot be changed.
Constructing Strings
There are several ways to create a String
in Java:
"Hello World!"
. New Instance: You can create a new instance of the String
class using the new
keyword, like String myString = new String("Hello");
. Using the ValueOf Method: You can use the valueOf
method to create a String
from a primitive value or an object, like String myString = String.valueOf(10);
.
Methods and Operations
Here are some common methods and operations you can perform on String
objects:
length()
method. Concatenation: You can concatenate two strings using the +
operator, like "Hello " + "World!"
. Substring: Extracts a substring from the original string using the substring(int beginIndex)
method. Replace: Replaces a portion of the string with another using the replace(String target, String replacement)
method. Split: Splits a string into an array of substrings based on a specified separator using the split(String regex)
method.
Example Code
Here's some example code that demonstrates how to work with String
objects:
public class Main {
public static void main(String[] args) {
// Create a string literal
String greeting = "Hello World!";
System.out.println(greeting); // Output: Hello World!
// Use the + operator for concatenation
String myName = "John";
String message = "Hello, " + myName;
System.out.println(message); // Output: Hello, John
// Extract a substring
String phrase = "The quick brown fox jumps over the lazy dog.";
String extracted = phrase.substring(0, 10);
System.out.println(extracted); // Output: The quick
// Replace a portion of the string
String text = "The cat is sleeping.";
String newText = text.replace("cat", "dog");
System.out.println(newText); // Output: The dog is sleeping.
// Split a string into substrings
String sentence = "This, is, a, test";
String[] split = sentence.split(",");
for (String s : split) {
System.out.println(s);
}
// Output:
// This
// is
// a
// test
}
}
In this example, we create a string literal and use it in concatenation, substring extraction, replacement, and splitting.
I hope this helps! Let me know if you have any questions.