How to assign a new value to a variable in java example
How to assign a new value to a variable in java example
I'm happy to respond in English for you!
In Java, variables are used to store values. Assigning a new value to a variable is a fundamental concept in programming. Here's an example of how to do it:
Let's say we have a variable x
and its initial value is 5:
int x = 5;
To assign a new value to x
, you can use the assignment operator (=). For example, let's change the value of x
to 10:
x = 10;
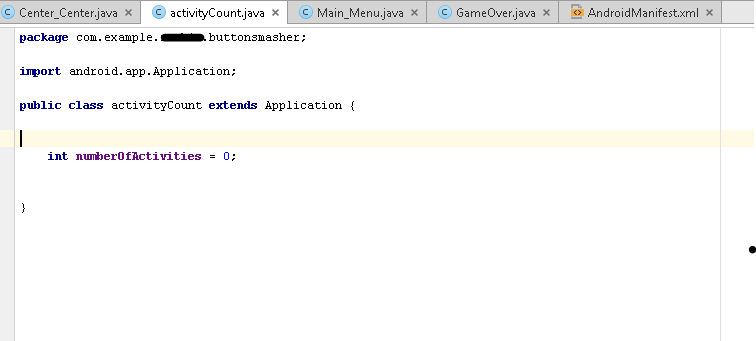
After this statement, the value of x
will be 10.
Here are some more examples:
Assigning a new integer value:int x = 5;
x = 20; // x is now 20
Assigning a new string value:
String name = "John";
name = "Jane"; // name is now "Jane"
Assigning a new boolean value:
boolean isAdmin = true;
isAdmin = false; // isAdmin is now false
Note that in Java, you can assign a new value to a variable only if the type of the new value matches the declared type of the variable. For example, you cannot assign an integer value to a String variable, and vice versa.
Here's a more complex example:
int x = 5;
if (x > 10) {
x = 15; // x is now 15
} else {
x = 20; // x is now 20
}
In this example, we use an if
statement to check the value of x
. If the condition is true, we assign a new value (15) to x
. Otherwise, we assign another value (20). The final value of x
will be determined by the outcome of the if
statement.
In summary, assigning a new value to a variable in Java involves using the assignment operator (=) with the variable name and the desired value. Make sure to match the type of the new value with the declared type of the variable.
How to assign a new value to a variable in java using
I'd be happy to help!
In Java, assigning a new value to a variable is quite straightforward. There are several ways to do this, depending on the context and the type of variable you're working with. Here's a breakdown:
Declaring and Assigning a Variable
When declaring a variable in Java, you can also assign it an initial value using the assignment operator (=). For example:
int myAge = 30; // declare and assign int variable
In this case, myAge
is assigned the value 30. The type of the variable (in this case, int
) must be specified before the name of the variable.
Updating a Variable
Once a variable has been declared and initialized, you can update its value using the assignment operator (=). For instance:
int myAge = 30;
myAge = 31; // update myAge to 31
In this example, we first declare and initialize myAge
with the value 30. Then, we assign a new value (31) to it using the assignment operator (=).
Using the += Operator for Compound Assignment
Java provides a shorthand way of updating variables called compound assignment operators. One common example is the += operator, which adds the right-hand operand to the left-hand operand and assigns the result back to the original variable.
Here's an illustration:
int myAge = 30;
myAge += 1; // equivalent to myAge = myAge + 1; -> updates myAge to 31
In this example, myAge
is initially set to 30. The += operator adds 1 to the current value of myAge
, effectively incrementing it by 1. The result (31) becomes the new value of myAge
.
Using Other Compound Assignment Operators
Java also supports other compound assignment operators, such as -=, *=, /=, %=, etc. These can be used in a similar fashion to update variables.
For instance:
int myAge = 30;
myAge -= 2; // equivalent to myAge = myAge - 2; -> updates myAge to 28
Here, the -= operator subtracts 2 from the current value of myAge
, resulting in a new value (28) for it.
Using the = Operator with Conditional Statements
Finally, you can use the assignment operator (=) within conditional statements, such as if-else or switch-case constructs. For example:
int myAge = 30;
if (myAge < 40) {
myAge = 35; // update myAge to 35 if it's less than 40
} else {
myAge = 45; // assign 45 to myAge if it's not less than 40
}
In this example, we're using an if-else statement to conditionally update the value of myAge
. The assignment operator (=) is used within the statement to set new values based on specific conditions.
These are some basic ways to assign new values to variables in Java. Of course, there's more to learn about Java syntax and best practices, but this should give you a solid foundation!